REST/JSON API¶
Cloud settings and usage¶
In order to use and test API, you have to meet these points:
The POD
icc-servicerest
is running in the KubernetesThere are set valid credentials for the user you are using to access API. You can create them using ReactAdmin.
API user has to have assigned the
RestRpc
roleThe “Swagger” (utility for testing the API) has to be enabled in the
icc-servicerest
POD
Integration¶
REST/JSON integration facilitates data import and export, both on an item-by-item and bulk basis. The interface is integrated in the WebAdmin administration web.
Solution Overview¶
Architecture¶
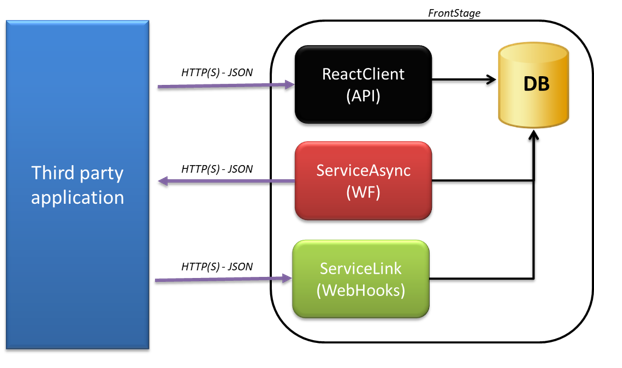
Fig. 1: Block diagram illustrating communication between FrontStage and an external application
The solution consists of the following components:
A third-party application
WebAdmin – A FrontStage module that passively receives, checks and executes requests from the external application for changes of data in the database.
ServiceAsync – A FrontStage module that runs workflows based on system events (entity status changes, time) and that actively sends requests to the external application.
DB – This is the iCC database accessed by all other modules.
The authentication method is determined by the IIS settings for the WebAdmin module; usually, WindowsIntegrated is used, while in heterogeneous environments it is possible to use, for instance, Basic (preferably with SSL).
The authorization method is determined by the FrontStage system rights, i.e. the Role/Permission/Scope settings.
HTTP(S) passive – receiving data¶
Integrated active operations for each of the entity types using prepared POCO structures:
Entity |
Create / POST |
Update / PUT |
Read / GET |
Delete / DELETE |
---|---|---|---|---|
Contact + PhoneNumber + Form |
all fields |
all fields |
all fields |
delete |
Issue + Form |
all fields |
all fields |
all fields |
close |
OutboundCall + Form* |
all fields |
information fields and the form |
all fields |
cancel until executed |
Message + Form* |
all fields |
information fields and the form |
all fields |
cancel until executed |
Entities |
N / A |
N / A |
all fields |
N / A |
Form** |
all fields |
all fields |
all fields |
Cancel |
* Works with multiple forms.
** Forms are transferred always as a key-value glossary structure since they are dynamically configurable; all values are transferred as strings.
The coding used is always UTF-8.
Operation results are primarily indicated with HTTP status codes:
200 – OK
201 – OK, created
204 – OK, without a return value
404 – The item does not exist
401 – Insufficient rights
422 – Data cannot be inserted in the DB (field length, unknown keys, limitations only in the DB etc.)
400 – A non-parsed item or an incorrect register
405 – The http method not supported; a mandatory URL parameter is missing; data inconsistency)
412 – The operation (PUT, POST, DELETE) is not possible due to the item status
415 – The media is not an application/json and the method is different than GET
HTTP(S) active – sending data¶
Reverse integration uses the possibilities of action triggers and asynchronous workflows. A node that sends data can be used in a workflow (WF) instance.
Note: Apart from the relevant section below, you can find more details on the use of workflows in “iCC – WF Programming”.
Entity |
WF node |
---|---|
Contact |
PostContact |
Issue |
PostIssue |
OutboundCall |
PostOutboundCall |
Message |
PostMessage |
Entities |
PostEntities |
Form |
PostForm |
Entity |
Trigger |
Event in UI |
---|---|---|
Contact |
ContactSaved |
New, Save, Delete |
Issue |
IssuePhaseChanged |
Change phase when saving |
IssueAssigned |
Change, assign agent when saving |
|
IssueUnassigned |
Remove agent when saving |
|
OutboundCall |
OutboundCallResultChanged |
New, Save, Call processing, Call result |
OutboundCallPhaseChanged |
Call processing |
|
Message |
MessageResultChanged |
New, Save, Message processing, Message result |
MessagePhaseChanged |
Message processing |
|
Form |
FormSaved |
Create, Save |
Security¶
All text values handed over from the outside to FrontStage through the REST interface (PUT, POST) can be scanned for the presence of any malicious code (XSS). This is controlled by the XssRestApiSet configuration parameter, which can have the following values:
None – No filtering of input strings
Filter – Filter usual undesirable HTML structures using blacklisting (HtmlSanitizer)
Protect – Filter all active HTML structures (AntiXSS)
Strict – Filter basic undesirable HTML structures and recode them into safe strings (HtmlCleanser+HtmlEncode)
All text values submitted outside of FrontStage through the REST interface (GET) and by means of WF (PostContact, PostIssue, PostOutboundCall, PostMessage) can be scanned for the presence of a malicious code (XSS). This is controlled by the XssRestApiGet configuration parameter.
Data structures¶
Data structures in the JSON format are used to transmit information. These structures have a fixed scheme, and it is known in advance except for the Data field in FormRest, which reflects the scenario definition and is user defined. This field contains a single object with properties of the text string type.
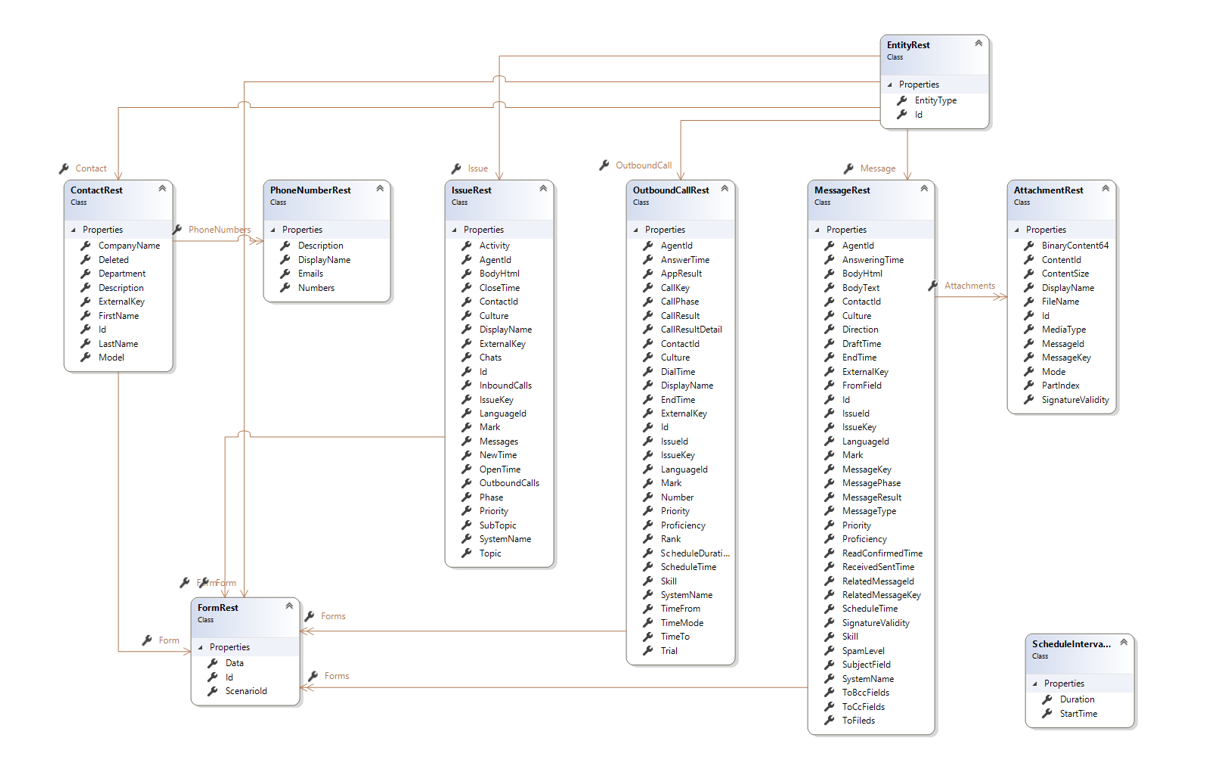
Fig. 2: Overview of data structures for the REST data exchange
Contacts¶
Processing contact data¶
When REST operations are performed with contacts (Contact) that change the contact value, the ContactSaved triggers are not called.
The required authorization is EditContact, for GET – AllowRead, and for PUT/POST/DELETE – AllowFull.
Reading an item¶
GET - Rest.aspx?Id=GUID [returns the ContactRest structure]
GET - Rest.aspx?ExternalKey=EXTERNALKEY [returns the ContactRest structure]
Pro Id=GUID, if the item exists but is Deleted, it is returned with 410
Reading an item array¶
GET - Rest.aspx?Skip=10&Take=20&Model=ABC&With=Numbers [returns the ContactRest array]
PhoneBoookId optional parameter – It returns only contacts from this PB up to max. 10,000 items; if a model is specified, then it filters through this model. If With is specified, it filters by the value – Numbers – having PN with numbers (returns numbers only); Emails – having PN with e-mails (returns e-mails only); NumbersOrEmails – having PN with e-mails or numbers (returns everything); None – having no PN, no definition or All – returns everything.
Editing an item¶
PUT - Rest.aspx?Id=GUID [requires the ContactRest structure; returns nothing]
204 – OK
201 – The item existed but was Deleted; it is now restored
If Deleted=true is entered, the item will be deleted.
Creating or editing an item¶
PUT - Rest.aspx?ExternalKey=EXTERNALKEY&Model=ABC [requires the ContactRest structure; returns the item GUID]
200 – OK
If Deleted=true is entered, the item will be deleted if it existed, otherwise nothing.
Items cannot be created without a model in the URL (in then returns 405).
If ExternalKey in URL is specified, than this contact is searched and if not found 404 error is returned. ExternalKey in ContactRest is not used for search, only as data input.
Creating an item¶
PUT - Rest.aspx&Model=ABC [requires the ContactRest structure; returns the item GUID]
201 - OK
If Deleted=true is entered, nothing will happen.
Items cannot be created without a model in the URL (in then returns 405)
Deleting an item¶
DELETE Rest.aspx?Id=GUID [returns nothing]
204 – OK
404 – The item does not exist.
Deletion can be performed only by setting Deleted=1
DELETE Rest.aspx?ExternalKey=EXTERNALKEY [returns the deleted item’s GUID]
200 – OK
404 – The item does not exist.
Deletion can be performed only by setting Deleted=1
Deletion is performed without cascading.
Editing an item set¶
POST Rest.aspx&Model=ABC [requires the ContactRest structure array, and returns an int array of error-free items
Items cannot be created without a model in the URL (405).
The operations performed are SYNC depending on the existence of the Id or ExternalKey and Deleted => Add/Update/Delete
Sending contact data¶
The PostContact WF node makes it possible to send the ContactRest structure for a contact with the InstanceRefId using POST to an external system and, if relevant, process the returned data. The ContactSaved trigger is called when saving or deleting the contact in the UI editors.
Data structures for Contact¶
Data structures are described as C# classes. Items highlighted with italics are always filled out in GET operations.
ContactRest¶
Name |
Length |
Meaning |
---|---|---|
FirstName* |
60 |
First name |
LastName* |
80 |
Family name |
CompanyName* |
120 |
Company name |
Department* |
60 |
Department name |
Description* |
300 |
Description |
ExternalKey |
48 |
This is an optional external key of the contact that is used for synchronization with external data sources. The key uniqueness is not checked during batch processing; nevertheless, if one and the same key is used multiple times, then one and the same record will be overwritten. |
Id |
This is an internal key of the contact (GUID). The key uniqueness is not checked during batch processing; nevertheless, if one and the same key is used multiple times, then one and the same record will be overwritten. If a non-existing key is entered, a new record will be created. If both Id and ExternalKey are entered, then Id has priority when the system checks whether the record exists. If neither Id nor ExternalKey is entered, a new record is always created; however, we do not recommend using it this way since an external application will have no possibility to refer to and work with the created records. |
|
Deleted |
Indicates that the contact is to be deleted. If the contact is deleted, it is marked as deleted, yet the telephone numbers and form data associated with it remain valid. If an external application subsequently asks for a contact to be created with the same ExternalKey or Id that one of the deleted contacts had, the contact will be recycled, i.e. only the indication of deleted will be removed and the submitted fields will be updated. |
|
PhoneNumbers |
This is a field with structures of telephone numbers and e-mail addresses associated with the contact. |
|
Form |
This is a FormRest structure with data from the associated data form. The form structure is determined by the contact model (Model in URL). If the form data field is not specified at all, yet it is included in the form structure, it will not be changed. If the form data field is to be set to NULL, it must be stated with the value of NULL. |
|
Forms |
This is an ID of forms associated with the cpntact. It is entered for the GET operations, else it is ignored. |
|
Model |
8 |
This is a name of the contact model. It is only stated when reading; it is ignored when writing. |
* The meanings and names of these fields are configured using the ContactModel table and can be changed. Therefore, the field needs to be understood rather as a generally usable field. The field can be filled out even if it is indicated as hidden in the ContactModel table.
public class ContactRest
{
public string FirstName { get; set; }
public string LastName { get; set; }
public string CompanyName { get; set; }
public string Department { get; set; }
public string Description { get; set; }
public string ExternalKey { get; set; }
public PhoneNumberRest[] PhoneNumbers { get; set; }
public FormRest Form { get; set; }
public bool Deleted { get; set; }
public Guid? Id { get; set; }*
public *\ *string*\ * Model { get; set; }*
}
PhoneNumberRest¶
Name |
Length |
Meaning |
---|---|---|
DisplayName |
150 |
Name |
Description |
50 |
Description |
Numbers |
80 |
This is a telephone number. When imported, the number is normalized according to the set numbering plan (typically a zero is added before the number). |
Emails |
250 |
This is an e-mail address. It is used literally when imported. |
Note: For the purposes of updating, the PhoneNumber record needs to be understood as if it had a key determined by the Numbers and the Emails fields for the given contact. If these fields match, the update is performed; if they do not match, the relevant value is inserted. If the given pair is not stated for the given contact during the update, it is deleted from the database for the given contact if it existed before (or it is marked as deleted). If such a pair is subsequently added, then the deleted record is recycled (for the given contact), i.e. the indication of deletion is removed and the submitted fields are updated. For these reasons, we recommend using exclusively either the Numbers field or the Emails field.
public class PhoneNumberRest
{
public string DisplayName { get; set; }
public string Description { get; set; }
public string Numbers { get; set; }
public string Emails { get; set; }
}
Data example¶
ContactRest structure array:
[
{
"CompanyName": "Systems, s s.r.o.",
"Deleted": false,
"ExternalKey": "R2f7b1468"
},
{
"CompanyName": "Motory a.s.",
"Department": "0030005",
"PhoneNumbers": [
{
"DisplayName": "Fixed",
"Numbers": "0271004242"
}
],
"Deleted": false,
"ExternalKey": "Rf3fd7a4"
},
{
"CompanyName": "ETNA spol. s.r.o.",
"Department": "43969757",
"PhoneNumbers": [
{
"DisplayName": "Fixed",
"Numbers": "094242"
}
],
"Form": {
"Data": {
"Subject": "Sale",
"A4": null,
"MemberNumber": "",
"PreferredContact": null,
"BaggageNo": "",
"Color": null,
"Remark": "",
"Delivery": null,
"Solution": "refund;exchange",
"Remarks": ""
}
},
"Deleted": false,
"ExternalKey": "R5351a13b"
}
]
or
[
{
"CompanyName": "ETNA spol. s.r.o. ",
"Department": "49969757 ",
"PhoneNumbers": [
{
"DisplayName": "Fixed",
"Numbers": "0271004242"
}
],
"Form": {
"Data": {
"Subject": "Sale",
"A4": null,
"MemberNumber": "9999",
"PreferredContact": null,
"BaggageNo": "11588",
"Color": null,
"Remark": "",
"Delivery": null,
"Solution": "refund;exchange",
"Remarks": "New remark !"
}
},
"ExternalKey": "KOMB54512"
}
]
{
"CompanyName": "AGRIC, s.r.o.",
"Department": "445452952",
"PhoneNumbers": [
{
"DisplayName": "Mobil",
"Numbers": "0602330533"
},
{
"DisplayName": "Pevná",
"Numbers": "0271004004"
}
],
"Deleted": false,
"Id": "c9f6103d-b57c-4c69-91b8-0e5d6e2f24b0",
"Model": "Company",
"Forms": [
"6fd600fc-fcaa-e711-8bc1-40b034090009",
"7cd600fc-fcaa-e711-8bc1-40b034090009",
"5e3d6653-fdaa-e711-8bc1-40b034090009"
]
}
Issues¶
Processing issue data¶
During the REST operations with issues (Issue), which change the issue phase (the Phase field), the IssuePhaseChanged trigger is called. Analogously, when the agent assigned to the issue is changed (AgentId/SystemName), the triggers of IssueAssigned and IssueUnassigned are used.
The required right is EditIssue, for GET – AllowRead, for PUT/POST/DELETE – AllowFull.
Reading an item¶
GET - Rest.aspx?Id=guid [returns the IssueRest structure]
GET - Rest.aspx?IssueKey=ISSUEKEY [returns the IssueRest structure]
Reading an item array¶
GET - Rest.aspx?Skip=10&Take=20&ProjectId=guid&Date=DDMMYYYY [returns the IssueRest array]
If ProjectId is entered, it returns just issues from the given project up to maximum 1000 items.
If Date is entered, it returns just issues with the record creation date (NewTime).
Either ProjectId or Date must always be entered, or both.
Editing an item¶
PUT - Rest.aspx?Id=guid [requires the IssueRest structure; returns nothing]
204 – OK, edited
405 – Both Activity and Phase are filled out, yet these values do not match.
Culture can be entered instead of LanguageId.
ExternalKey can be entered instead of ContactId.
SystemName can be entered instead of AgentId.
Relevant rules are observed when editing Phase; if the required phase is inconsistent with the rules, the phase is not changed (Error 412).
If SubTopic does not match Topic, then the change is not made (Error 405).
If both Phase and Topic or SubTopic are changed during editing, then Phase is changed first and only then Topic/SubTopic.
Creating or editing an item¶
PUT - Rest.aspx?IssueKey=ISSUEKEY&ProjectId=guid [requires the IssueRest structure; returns the item’s GUID ]
200 – OK, edited
201 – OK, created
405 – Both Activity and Phase are entered, yet these two values do not match.
Items cannot be created without a ProjectId in the URL (Error 405).
Culture can be entered instead of LanguageId.
ExternalKey can be entered instead of ContactId.
SystemName can be entered instead of AgentId.
If IssueRest does not have IssueKey entered, the value from the URL will be used instead.
The Phase field is ignored when an item is created, and rules are applied.
Relevant rules are observed when editing Phase; if the required phase is inconsistent with the rules, the phase is not changed (Error 412).
If SubTopic does not match Topic, then the change is not made (Error 405).
If both Phase and Topic or SubTopic are changed during editing, then Phase is changed first and only then Topic/SubTopic.
If IssueKey in URL is specified, than this issue is searched and if not found 404 error is returned. IssueKey in IssueRest is not used for search, only as data input.
Creating an item¶
PUT - Rest.aspx&ProjectId=guid [requires the IssueRest structure; returns the item’s GUID]
201 – OK, created
405 – Both Activity and Phase are entered, yet these two values do not match.
Items cannot be created without a ProjectId in the URL (Error 405).
Culture can be entered instead of LanguageId.
ExternalKey can be entered instead of ContactId.
SystemName can be entered instead of AgentId.
The Phase field is ignored when an item is created, and rules are applied.
If SubTopic does not match Topic, then the record is not created (Error 412).
Creating or editing an item set¶
POST Rest.aspx&ProjectId=guid [requires the IssueRest structure field; returns an array of int items that are free of error]
Items cannot be created without a ProjectId in the URL (Error 405).
Culture can be entered instead of LanguageId.
ExternalKey can be entered instead of ContactId.
SystemName can be entered instead of AgentId.
The Phase field is ignored when an item is created, and rules are applied.
Relevant rules are observed when editing Phase; if the required phase is inconsistent with the rules, the phase is not changed (Error 412).
If SubTopic does not match Topic, then the change is not made (Error 405).
If both Phase and Topic or SubTopic are changed during editing, then Phase is changed first and only then Topic/SubTopic.
Note: Since the API fully processing the logic of Issues for every item (triggers, rule verification etc.), it is necessary to verify on a particular installation what the reasonable upper limit is for the number of items processed simultaneously.
Closing an item¶
DELETE - Rest.aspx?Id=guid [closes a record; returns nothing]
204 – OK
404 – The item does not exist.
The item will be closed by setting Activity=Closed and the default phase for this activity; the rules of transition between phases are ignored.
405 – No default phase for closure defined.
412 – The issue has already been closed.
DELETE - Rest.aspx?IssueKey=ISSUEKEY [returns the canceled record’s GUID]
200 – OK
404 – The item does not exist.
The item will be closed by setting Activity=Closed and the default phase for this activity; the rules of transition between phases are ignored.
405 – No default phase for closure defined.
412 – The issue has already been closed.
Forced setting of issue phase¶
For the PUT and POST operations, it is possible to add the ForcePhase parameter in the URL with the value of “true”. In that case, the Phase parameter is required, which is used to set the PhaseId and Activity fields of the issue. No check is performed of the allowed transitions between phases using PhaseTransition.
When forced phase setting is used, the system does not generate any IssuePhaseChanged triggers.
Recommendation: Since the phase is selected based on the Phase.Rank field, it is necessary that the Rank value in the register be globally unique regardless of Topic/Subtopic.
Important: The forced phase setting can be used to set such a combination of the Topic/Subtopic/Phase fields that is not allowed during phase transition (PhaseTransition), which can lead the user to a dead end where they will not be able to change the issue phase.
Example: PUT - Rest.aspx?Id=guid&ForcePhase=true [requires the IssueRest structure; returns nothing]
Sending issue data¶
The WF PostIssue node allows the IssueRest structure of the issue to be sent with InstanceRefId using POST to an external system and, if relevant, process any returned data. The IssuePhaseChanged trigger is called when the issue phase changes. The IssueAssigned trigger is called when an agent is assigned to the issue, IssueUnassigned when the agent is removed from the issue.
Data structures for Issues¶
Data structures are described as C# classes. Items highlighted in italics are always filled out in GET operations.
IssueRest¶
Name |
Length |
Meaning |
---|---|---|
Activity |
32 |
Indicates the issue status (a generalized phase). It takes one of the following values:
When a new record is created, the field is ignored and the status of “New” is always used. It is recommended that you enter either this field or the Phase field, yet not both at the same time since the fields are connected through the Phase register, and if the connection is inconsistent with the entered combination, the system will return Error 405. |
Phase |
This is the issue processing phase based on the Phase register of the Rank field. If it is left out or NULL, the data is not changed. This field is ignored when a new record is created. |
|
Topic |
This is the issue topic based on the Topic register of the Rank field. If it equals -1, the data is deleted. If it is left out or NULL, the data is not changed. |
|
SubTopic |
This is the issue subtopic based on the SubTopic register of the Rank field. If it equals -1, the data is deleted. If it is left out or NULL, the data is not changed. |
|
DisplayName |
320 |
This is optional text field. |
BodyHtml |
2GB |
Optional HTML formatted text of message. It will subject to XSS screening. |
AgentId |
This is an internal key (GUID) of the agent to whom the issue is assigned. If it contains “00000000-0000-0000-0000-000000000000”, the Agent field is deleted. If it does not exist or is NULL, the data is not changed. If the key is not found in the AgentId table, Error 400 occurs. |
|
SystemName |
200 |
This is a system login name of the agent to whom the issue has been assigned. If it is stated as AgentId, then SystemName is used. If it contains an empty string “”, the Agent field is deleted. If it does not exist or is NULL, the data is not changed. If the key is not found in the AgentId table, Error 400 occurs. |
Priority |
This is a value from 0 to 100 that indicates issue priority (100 being the highest). |
|
Mark |
An application mark; an integer from -2147483647 to 2147483647. |
|
LanguageId |
This is an internal key (GUID) of the language in which the issue is to be managed. If it contains “00000000-0000-0000-0000-000000000000”, the field is deleted. If it is specified, then it must be one of the keys in the Languages table, otherwise Error 400 occurs. |
|
Culture |
This is a two-letter ISO code of the language in which the issue is to be managed. If it is specified, it must be one of the keys in the Languages table, otherwise Error 400 occurs. If it contains an empty string “”, the Language field is deleted. If it is stated as Culture as well as LanguageId, then Culture is used. |
|
ContactId |
This is an internal key (GUID) of the contact with which the issue is to be associated. If the key does not exist, Error 400 occurs. |
|
ExternalKey |
48 |
This is an optional external key of the contact with which the issue is to be associated. If both ContactId and ExternalKey are specified, then ExternalKey is used. If it contains an empty string “”, the Contact field is deleted. If a non-existing ExternalKey is entered, Error 400 occurs. |
Form |
This is the FormRest structure with data of the associated data form. The form scheme depends on the topic and subtopic (specifically on the FormScenarioId fields of the Topic and the SubTopic registers). If the form data field is not specified at all but it is included in the form scheme, it will not be changed. If the form data field is to be set to NULL, it must be stated with the value of NULL. |
|
IssueKey |
48 |
This is an optional external key of the issue that is used for synchronization with external data sources. The key uniqueness of the key during batch processing is not checked. |
Id |
This is an internal key (GUID) of the issue. It is entered for the GET operations, else it is ignored. |
|
NewTime |
This is the issue creation time. It is entered for the GET operations, else it is ignored. |
|
OpenTime |
This is the issue opening time. It is entered for the GET operations, else it is ignored. |
|
CloseTime |
This is the issue closing time. It is entered for the GET operations, else it is ignored. |
|
OutboundCalls |
This is an ID of outbound calls associated with the issue. It is entered for the GET operations, else it is ignored. |
|
InboundCalls |
This is an ID of inbound calls associated with the issue. It is entered for the GET operations, else it is ignored. |
|
Messages |
This is an ID of messages associated with the issue. It is entered for the GET operations, else it is ignored. |
|
Forms |
This is an ID of forms associated with the issue. It is entered for the GET operations, else it is ignored. |
|
ProjectId |
ProjectID (GUID) of given issue. Filled during GET. |
|
ForcePhase |
Indicates a change in the phase control mode:
|
public class IssueRest
{
public string Activity { get; set; }
public int? Phase { get; set; }
public int? Topic { get; set; }
public int? SubTopic { get; set; }
public string DisplayName { get; set; }
public string BodyHtml { get; set; }
public Guid? AgentId { get; set; }
public string SystemName { get; set; }
public int? Priority { get; set; }
public int? Mark { get; set; }
public Guid? LanguageId { get; set; }
public string Culture { get; set; }
public Guid? ContactId { get; set; }
public string ExternalKey { get; set; }
public FormRest Form { get; set; }
public Guid? Id { get; set; }*
public string IssueKey { get; set; }
public DateTime? NewTime { get; set; }*
public DateTime? OpenTime { get; set; }*
public DateTime? CloseTime { get; set; }*
public Guid[] OutboundCalls { get; set; }*
public Guid[] InboundCalls { get; set; }*
public Guid[] Messages { get; set; }*
}
Example of data¶
IssueRest structure:
{
"Activity": "New",
"Topic": 160,
"SubTopic": 80,
"Priority": 0,
"Mark": 0,
"Form": {
"Data": {
"From": "Sadil",
"Anonymous": "False",
"Body": "Please indicate when bicycles can be transported to Pilsen",
"ResponseType": "E",
"Authorization": null,
"RespEmail": "sadil@atlantis.cz",
"RespAddressStreet": "Street address 1234",
"RespAddressCity": "Sedlčany",
"RespAddressZIP": "26401",
"VecHled": null
},
"ScenarioId": "1b22b0cd-345a-490b-9260-e8274f4e1111",
"Id": "41f3ba16-cf1b-e611-885d-9439e5913128"
},
"Id": "40f3ba16-cf1b-e611-885d-9439e5913128",
"NewTime": "2016-04-01T13:37:39.837",
"OutboundCalls": [],
"InboundCalls": [],
"Messages": [
"28420271-d11b-e611-885d-9439e5913128",
"7a0e4e9d-d11b-e611-885d-9439e5913128"
]
}
{
"Activity": "Closed",
"Phase": 20,
"Topic": 90,
"DisplayName": "Nadpis",
"BodyHtml": "<p>Tak <span style=\\"font-family: Lucida Sans Unicode,Lucida Grande,sans-serif;\\"><strong>co </strong></span>?</p>",
"AgentId": "4354b36d-e2ab-46ad-bc78-347edcd9b293",
"SystemName": "atlantis2000\\\\zidek",
"Priority": 2,
"Mark": 0,
"LanguageId": "632a5e30-29c1-4ed1-a3ec-c494f3016817",
"Culture": "en",
"Id": "04c2ae2d-4ffc-e511-881c-9439e5913128",
"IssueKey": "XS122",
"NewTime": "2016-04-07T01:27:52.857",
"OpenTime": "2016-04-14T15:20:09.273",
"CloseTime": "2018-07-24T03:14:03.933",
"OutboundCalls": [
"00c2ae2d-4ffc-e511-881c-9439e5913128"
],
"InboundCalls": [
"1ed47e69-0e52-e811-8be9-40b034090009",
"dcb01f4b-1052-e811-8be9-40b034090009",
"cbb5475d-1052-e811-8be9-40b034090009",
"4dbd6feb-cd8e-e811-8c00-40b034090009",
"d48cb73f-d98e-e811-8c00-40b034090009",
"8ab9becf-de8e-e811-8c00-40b034090009"
],
"Messages": [
"bd825d50-020d-e711-8a3d-9439e5913128"
],
"Chats": [],
"ProjectId": "18e001d5-a6c7-426c-988d-3fbfedbc88b2"
}
Outbound calls¶
Outbound calls are uniquely identified only using their Id. When CallKey is used, the last call with the given CallKey is always considered. The reason behind is a mechanism how FrontStage processes failed calls (e.g. if the line is busy): It creates a new record with a new Id, yet with the same CallKey.
Processing call data¶
During REST operations with outbound calls (OutboundCall) that change the call status (the CallResult field), the OutboundCallResultChanged triggers are called.
The required authorization is EditOutboundCall, for GET it is AllowRead, and for PUT/POST/DELETE it is AllowFull.
Reading an item¶
GET - Rest.aspx?Id=guid&ScenarioId=guid [returns the OutboundCallRest structure]
GET - Rest.aspx?CallKey=CALLKEY&ScenarioId=guid [returns the OutboundCallRest structure]
If ScenarioId is specified, only forms according to the given scenario are returned, else all forms are returned.
If there are multiple calls with the given CallKey (multiple call attempts), the record of the last attempt is returned (with the highest Trial and the latest time).
Reading an item array¶
GET - Rest.aspx?Skip=10&Take=20&OutboundListId=guid&ScenarioId=guid&Date=DDMMYYYY [returns the OutboundCallRest array]
If OutboundListId is specified, it returns just calls from this outbound campaign up to max. 1000 items.
If Date is specified, it only lists calls with the given date of record creation (TimeUtc).
Either OutboundListId or Date must always be specified, alternatively both.
If ScenarioId is specified, only forms based on the given scenario are returned, else all forms are returned.
Editing an item¶
PUT - Rest.aspx?Id=guid&ScenarioId=guid&Schedule=ListOnly [requires the OutboundCallRest structure, returns nothing]
204 – OK, edited
412 – The current call status (CallResult) is not Prepared, Scheduled, Paused.
405 – CallResult is specified, yet it has a different value than the allowed values of Scheduled, Paused, Canceled, Neglected.
409 – The call is subject to scheduling, yet there is no free capacity for the required combination of ScheduleTime+ScheduleDuration – the server returns a JSON field of structures of the ScheduleIntervalRest type with possible alternative periods when the call could be created and scheduled. This error can occur during item creation or during editing.
If the Forms field is filled out, then ScenarioId must be specified in the URL. If there is a form based on such a ScenarioId, it is edited, otherwise a new form is created. If there are multiple forms with the given ScenarioId, the form instance ID in the FormRest structure is considered; if no ID is specified in FormRest, the first one found according to the ScenarioId will be edited (which need not be a desirable behavior).
Culture can be entered instead of LanguageId.
ExternalKey can be entered instead of ContactId.
IssueKey can be entered instead of IssueId.
SystemName can be entered instead of AgentId.
If an agent is specified, the call is set as pre-distributed.
ScenarioId is required only if forms are imported and these forms do not have any ScenarioId specified in the FormRest structure.
Schedule parameter is optional; it indicates the call scheduling mode if the calls are subject to capacity planning; the possible modes are the following:
ListOnly = The call should not be scheduled, just a list of free times should be returned (as if it is impossible to schedule the call – with 200 OK).
Normal = This is the default behavior; if scheduling is possible, the call will be scheduled (204 OK), otherwise a list of free times is returned (409 Conflict).
Forced = The call should be planned regardless of the scheduling rules (it returns 204 OK; it never returns a list of times).
Creating or editing an item¶
PUT - Rest.aspx?CallKey=CALLKEY&OutboundListId=guid&ScenarioId=guid&Schedule=ListOnly [requires the OutboundCallRest structure, returns the item’s GUID]
200 – OK, edited
201 – OK, created
412 – The current call status (CallResult) is not Prepared, Scheduled, Paused.
405 – CallResult is specified, yet it has a different value than the allowed values of Scheduled, Paused; Canceled or Neglected can also be used when editing; even Prepared when creating.
409 – The call is subject to scheduling, yet there is no free capacity for the required combination of ScheduleTime+ScheduleDuration – the server returns a JSON field of structures of the ScheduleIntervalRest type with possible alternative periods when the call could be created and scheduled. This error can occur during item creation or during editing.
If the Forms field is filled out, then ScenarioId must be specified in the URL. If there is a form based on such a ScenarioId, it is edited, otherwise or when an item is being created, a new form is created. If there are multiple forms with the given ScenarioId, the form instance ID in the FormRest structure is considered; if no ID is specified in FormRest, the first one found according to the ScenarioId will be edited (which need not be a desirable behavior).
Items cannot be created without an OutboundListId in the URL (Error 405).
Culture can be entered instead of LanguageId.
ExternalKey can be entered instead of ContactId.
IssueKey can be entered instead of IssueId.
SystemName can be entered instead of AgentId.
If an agent is specified, the call is set/created as pre-distributed.
ScenarioId is required only if forms are imported and these forms do not have any ScenarioId specified in the FormRest structure.
If OutboundCallRest has no CallKey specified, then a value from the URL will be used for it.
Schedule parameter is optional; it indicates the call scheduling mode if the calls are subject to capacity planning; the possible modes are the following:
ListOnly = The call should not be scheduled, just a list of free times should be returned (as if it is impossible to schedule the call – with 200 OK).
Normal = This is the default behavior; if scheduling is possible, the call will be scheduled (204 OK), otherwise a list of free times is returned (409 Conflict).
Forced = The call should be planned regardless of the scheduling rules (it returns 204 OK; it never returns a list of times).
If CallKey in URL is specified, than this call is searched and if not found 404 error is returned. CallKey in OutboundCallRest is not used for search, only as data input.
Creating an item¶
PUT - Rest.aspx&OutboundListId=guid&ScenarioId=guid [requires the OutboundCallRest structure, returns the item’s GUID]
201 – OK, created
405 –CallResult is specified, yet it has a different value than the allowed values of Prepared, Scheduled, Paused.
409 – The call is subject to scheduling, yet there is no free capacity for the required combination of ScheduleTime+ScheduleDuration – the server returns a JSON field of structures of the ScheduleIntervalRest type with possible alternative periods when the call could be created and scheduled. This error can occur during item creation or during editing.
If the Forms field is filled out, then ScenarioId must be specified in the URL. New forms are always created.
Items cannot be created without an OutboundListId in the URL (Error 405).
Culture can be entered instead of LanguageId.
ExternalKey can be entered instead of ContactId.
IssueKey can be entered instead of IssueId.
SystemName can be entered instead of AgentId.
If an agent is specified, the call is created as pre-distributed.
ScenarioId is required only if forms are imported; this method allows the importing only of forms with the same ScenarioId.
Schedule parameter is optional; it indicates the call scheduling mode if the calls are subject to capacity planning; the possible modes are the following:
ListOnly = The call should not be scheduled, just a list of free times should be returned (as if it is impossible to schedule the call – with 200 OK).
Normal = This is the default behavior; if scheduling is possible, the call will be scheduled (204 OK), otherwise a list of free times is returned (409 Conflict).
Forced = The call should be planned regardless of the scheduling rules (it returns 204 OK; it never returns a list of times).
Creating set of items¶
POST Rest.aspx?OutboundListId=guid&ScenarioId=guid [requires an array of the OutboundCallRest structures, returns an array of int items that are free of error]
Items cannot be created without an OutboundListId in the URL (Error 405).
Culture can be entered instead of LanguageId.
ExternalKey can be entered instead of ContactId.
IssueKey can be entered instead of IssueId.
SystemName can be entered instead of AgentId.
If an agent is specified, the call is created as pre-distributed.
ScenarioId is required only if forms are imported; this method allows the importing only of forms with the same ScenarioId.
Schedule parameter is optional; it indicates the call scheduling mode if the calls are subject to capacity planning; the possible modes are the following:
Normal = this is the default behavior; if scheduling is possible, the call will be imported, otherwise not imported.
Forced = the calls are imported without considering capacity planning rules.
Note: This method does not allow you to obtain a list of alternative periods for calls that are subject to capacity scheduling if scheduling of call fails due to a lack of capacity.
Canceling an item¶
DELETE - Rest.aspx?Id=guid [cancels a record, returns nothing]
204 – OK
404 – The item does not exist.
412 – The item is not in a status (CallResult) of Prepared, Paused or Scheduled and it cannot be changed anymore.
DELETE - Rest.aspx? CallKey=CALLKEY [returns the canceled record’s GUID]
200 – OK
404 – The item does not exist.
412 – The item is not in a status (CallResult) of Prepared, Paused or Scheduled and it cannot be changed anymore.
Sending outbound call data¶
The PostOutboundCall workflow node allows the OutboundCallRest structure for a call with an InstanceRefId to be sent using POST to an external system and, if relevant, process the returned data. The OutboundCallResultChanged and the OutboundCallPhaseChanged triggers are called when the outbound call result or phase changes.
Data structures for outbound calls¶
Data structures are described as C# classes. Items highlighted in italics are always filled out in GET operations.
OutboundCallRest¶
Name |
Length |
Meaning |
---|---|---|
Number |
32 |
A telephone number. When imported, the number is normalized based on the defined numbering plan (typically a 0 is added before the number). It is a required field. |
DisplayName |
120 |
A name. |
ScheduleTime |
This is the time for which the call is scheduled, expressed in local time. The call will not be distributed to agents before until this time. JSON format: “2016-06-27T19:15:01.9706909+02:00”. It is a recommended piece of data for calls that are subject to capacity scheduling; if this information is not specified for this type of calls, the current time will be used. |
|
ScheduleDuration |
This is the scheduled call duration in seconds. It is a recommended piece of data for calls that are subject to capacity scheduling; if this information is not specified for this type of calls, the duration of normal calls will be used, as specified for the call project (the NormalCallDuration parameter); if not even this parameter is specified, the duration of 120 seconds will be used. |
|
ScheduleBefore |
For calls that are subject to capacity scheduling, it is possible to specify for how long before the scheduled call time (ScheduleTime) the capacity availability is to be analyzed if the required time cannot be fulfilled. If this value is not specified, a pre-configured default value will be used (the OutCallScheduleBefore parameter). It specifies a shift in seconds and it is a positive number. |
|
ScheduleAfter |
For calls that are subject to capacity scheduling, it is possible to specify for how long after the scheduled call time (ScheduleTime) the capacity availability is to be analyzed if the required time cannot be fulfilled. If this value is not specified, a pre-configured default value will be used (the OutCallScheduleAfter parameter). It specifies a shift in seconds and it is a positive number. |
|
TimeMode |
16 |
This is a period of allowed call scheduling. If this field is specified, then it must indicate one of the following options:
If it contains an empty string “”, the fields TimeMode, TimeFrom and TimeTo are deleted. If the field contains anything else, the call is not inserted/changed. If the field does include one of the valid values, yet the TimeFrom or TimeTo field does not specify any time, the call is not inserted/changed. For calls that are subject to capacity scheduling, the TimeMode mechanism is ignored when the capacity and period availability are checked. If the call is subject to capacity planning, and selected TimeMode does not match its ScheduleTime, it won’t be automatically rescheduled. |
TimeFrom |
This is the local start time of the call scheduling condition of TimeMode. JSON format: “2016-06-27T19:15:01.9706909+02:00”. |
|
TimeTo |
This is the local start time of the call scheduling condition of TimeMode. JSON format: “2016-06-27T19:15:01.9706909+02:00”. |
|
Rank |
This is a positive integer (max. 2147483647) that specifies the call processing rank. If it is specified, then calls are distributed according to this rank. |
|
Skill |
This is a value from 0 to 100 that specifies a minimum skill of the agent to whom the call will be distributed. Calls are imported to the project specified for the given outbound campaign (OutboundListId). If no skill is explicitly stated, then the skill specified for the given outbound campaign is used (OutboundListId). |
|
LanguageId |
This is an internal key (GUID) of the language in which the call is to be managed. If it contains “00000000-0000-0000-0000-000000000000”, the field is deleted. If it is specified, then it must be one of the keys in the Languages table, otherwise the call is not inserted/changed. |
|
Culture |
This is a two-letter ISO code of the language in which the call is to be managed. If it is specified, it must be one of the keys in the Languages table, otherwise the call is not inserted/changed. If both Culture and LanguageId are stated, then Culture is used. If no language is explicitly stated, then the language specified for the given outbound campaign is used (OutboundListId), provided that it is at all specified. |
|
Proficiency |
This is a value from 0 to 100 that specifies a minimum language proficiency of the agent to whom the call will be distributed. If no proficiency is explicitly stated, then the proficiency specified for the given outbound campaign is used (OutboundListId), provided that it is at all specified. |
|
Priority |
This is a value from 0 to 100 that specifies the call priority (with 100 being the highest); calls with a higher priority are distributed earlier. If no priority is explicitly stated, then the priority specified for the given outbound campaign is used (OutboundListId), provided that it is at all specified. |
|
Absolute |
Flag marking the call to be distributed according to absolute time (EnqueuingTime) so it can be queued in similar way as inbound calls. Be sure that you have a mechanism how avoid overload by these calls (e.g. using TimeMode window). |
|
Mark |
This is an application mark; an integer from -2147483647 to 2147483647. |
|
ContactId |
This is an internal key (GUID) of the contact with which the call is to be associated. If it contains “00000000-0000-0000-0000-000000000000”, the Contact field is deleted. If the key does not exist or is NULL, the data is not changed. If this key is not found in the Contact table, then Error 400 occurs. |
|
ExternalKey |
48 |
This is an optional external key of the contact that with which the call is to be associated. If both ContactId and ExternalKey are specified, then ExternalKey is used. If it contains an empty string “”, the Contact field is deleted. If the key does not exist or is NULL, the data is not changed. If this key is not found in the Contact table, then Error 400 occurs. |
AgentId |
This is an internal key (GUID) of the agent to whom the call is to be assigned (using the pre-distribution mechanism). If it contains “00000000-0000-0000-0000-000000000000”, the Agent field is deleted. If the key does not exist or is NULL, the data is not changed. If this key is not found in the AgentId table, then Error 400 occurs. |
|
SystemName |
200 |
This is a system login name of the agent to whom the call is to be assigned (using the pre-distribution mechanism). If both AgentId and SystemName are specified, then SystemName is used. If it contains an empty string “”, the Agent field is deleted. If it does not exist or is NULL, the data is not changed. If the key is not found in the Agent table, Error 400 occurs. |
IssueId |
This is an internal key (GUID) of the case with which the call is to be associated. If it contains “00000000-0000-0000-0000-000000000000”, the Case field is deleted. If the key does not exist or is NULL, the data is not changed. If this key is not found in the Issue table, then Error 400 occurs. |
|
IssueKey |
48 |
This is an optional external key of the case with which the call is to be associated. If both IssueId and IssueKey are specified, then IssueKey is used. If it contains an empty string “”, the Case field is deleted. If the key does not exist or is NULL, the data is not changed. If this key is not found in the Issue table, then Error 400 occurs. |
CallKey |
48 |
This is an optional external key of the call, which is used for synchronization with external data sources. The key uniqueness is not checked during batch processing. |
Forms |
This is an array of FormRest structures with data from the associated data forms. The form scheme is determined by the ScenarioId parameter in the URL with the following effects:
|
|
Id |
This is an internal key (GUID) of the outbound call. It is specified for the GET operations, otherwise it is ignored. |
|
CallType |
16 |
This is the call type. It can contain the following values:
It is filled out during GET operations. This field can be set only when creating new record. It cannot be changed later. |
CallResult |
16 |
This is the call result (status). It can contain the following values:
It is filled out during GET operations. When making changes in PUT, it is allowed to make them only for calls in the following statuses: Prepared, Scheduled, Paused, with the allowed required value being Prepared, Scheduled, Paused, Canceled, Neglected. |
CallPhase |
16 |
This is the processing phase. It can contain the following values:
This is filled out during GET operations, otherwise it is ignored. |
CallResultDetail |
A detailed call result based on the CallResultDetail register of the Rank field. This is filled out during GET operations, otherwise it is ignored. |
|
AppResult |
80 |
An application call result – a free text. This is filled out during GET operations, otherwise it is ignored. |
Trial |
A call trial (1 – first). This is filled out during GET operations, otherwise it is ignored. |
|
DialTime |
This is the dial start time. It only applies to calls that reached the dialing phase. This is filled out during GET operations, otherwise it is ignored. |
|
AnswerTime |
This is the call answer time. It only applies to calls that reached the conversation phase. This is filled out during GET operations, otherwise it is ignored. |
|
EndTime |
This is the call end time. It only applies to terminated calls. This is filled out during GET operations, otherwise it is ignored. |
|
ProjectId |
ProjectID (GUID) of given call. Filled during GET. |
|
OutboundListId |
OutboundListID (GUID) of given call. Filled during GET. |
public class OutboundCallRest
{
public string Number { get; set; }
public string DisplayName { get; set; }
public DateTime? ScheduleTime { get; set; }
public int? ScheduleDuration { get; set; }
public int? ScheduleBefore { get; set; }
public int? ScheduleAfter { get; set; }
public string TimeMode { get; set; }
public DateTime? TimeFrom { get; set; }
public DateTime? TimeTo { get; set; }
public int? Rank { get; set; }
public int? Skill { get; set; }
public Guid? LanguageId { get; set; }
public string Culture { get; set; }
public int? Proficiency { get; set; }
public int? Priority { get; set; }
public bool? Absolute { get; set; }
public int? Mark { get; set; }
public Guid? ContactId { get; set; }
public string ExternalKey { get; set; }
public Guid? AgentId { get; set; }
public string SystemName { get; set; }
public FormRest[] Forms { get; set; }
public string CallKey { get; set; }
*public Guid? Id { get; set; }*
*public string CallResult { get; set; }*
*public string CallPhase { get; set; }*
*public int? CallResultDetail { get; set; }*
*public string AppResult { get; set; }*
*public int? Trial { get; set; }*
*public DateTime? DialTime { get; set; }*
*public DateTime? AnswerTime { get; set; }*
*public DateTime? EndTime { get; set; }*
*public Guid? ProjectId { get; set; }*
*public Guid? OutboundListId { get; set; }*
public Guid? IssueId { get; set; }
public string IssueKey { get; set; }
}
Data example¶
OutboundCallRest data structure:
{
"Number": "0602330533",
"ExternalKey": "KOMBO2",
"CallKey": "MYC113",
"IssueKey": "CASE-14451",
"Forms": [
{
"Data": {
"Subject": "SaleAA",
"A4": null,
"MemberNumber": "9999",
"PreferredContact": null,
"BaggageNo": "11588",
"Color": null,
"Remark": "",
"Delivery": null,
"Solution": "refund;exchange",
"Remarks": "New remark !"
}
}
]
}
{
"Number": "512",
"ScheduleTime": "2021-04-19T13:36:21.483",
"Skill": 75,
"LanguageId": "e358ec23-86d3-4dd1-90f5-2fd03ba714af",
"Culture": "cs",
"Proficiency": 60,
"Priority": 2,
"Mark": 0,
"AgentId": "4354b36d-e2ab-46ad-bc78-347edcd9b293",
"SystemName": "atlantis2000\\\\zidek",
"Id": "65e19a18-03a1-eb11-8bca-bce92ff829d5",
"CallType": "DialOut",
"CallResult": "NoResult",
"CallPhase": "HangupCalled",
"Trial": 1,
"DialTime": "2021-04-22T18:21:45.89",
"AnswerTime": "2021-04-22T18:21:54.917",
"EndTime": "2021-04-22T18:21:56.627",
"ProjectId": "18f26f7a-0c97-4a66-89a7-7299253c0a99",
"OutboundListId": "1de537f5-6767-4acc-8c32-63fb47c0f153",
"IssueId": "f60283d2-86a3-eb11-8bcd-bce92ff829d5"
}
ScheduleIntervalRest¶
Name |
Length |
Meaning |
---|---|---|
StartTime |
This is the free interval start time in local time. If the free interval in the database starts before the period defined by the ScheduleTime-ScheduleBefore, the first interval will begin with this time. JSON format: “2016-06-27T19:15:01.9706909+02:00”. |
|
Duration |
This is the free interval duration in seconds, ensuring that it includes possible call starts (ScheduleTime) while considering their planned duration (ScheduleDuration). |
public class ScheduleIntervalRest
{
public DateTime StartTime { get; set; }
public int Duration { get; set; }
}
Data example¶
ScheduleIntervalRest data structure:
{
"StartTime": "2016-06-27T19:15:01.9706909+02:00",
"Duration": 300
}
Messages¶
Messages are uniquely identified only using their Id. When MessageKey is used, the last message with the given MessageKey is always considered.
Processing message data¶
During REST operations with messages (Message) that change the message status (the MessageResult field), the MessageResultChanged triggers are called.
The required authorization is EditMessage, for GET it is AllowRead, and for PUT/POST/DELETE it is AllowFull. POST operations in active state as Receiving (for inbound), Draft, New or Scheduled (for outbound) requires only AllowWrite.
Reading an item¶
GET - Rest.aspx?Id=guid&ScenarioId=guid&WithBinary=true [returns the MessageRest structure]
GET - Rest.aspx?MessageKey=MSGKEY&ScenarioId=guid&WithBinary=true [returns the MessageRest structure]
If ScenarioId is specified, only forms according to the given scenario are returned, else all forms are returned.
If there are multiple messages with the given MessageKey, the record of the last record is returned (with the latest creation time).
If WithBinary=true is specified, then field BinaryContent64 is filled, otherwise only metadata of attachments are supplied.
Reading an item array¶
GET - Rest.aspx?Skip=10&Take=20&CampaignId=guid&ScenarioId=guid&Date=DDMMYYYY&WithBinary=true [returns the MessageRest array]
If CampignId is specified, it returns just messages from this outbound campaign up to max. 1000 items.
If Date is specified, it only lists messages with the given date of record creation (TimeUtc) up to max. 1000 items.
If WithBinary=true is specified, then field BinaryContent64 is filled, otherwise only metadata of attachments are supplied.
Either CampignId or Date must always be specified, alternatively both.
If ScenarioId is specified, only forms based on the given scenario are returned, else all forms are returned.
Editing an item¶
PUT - Rest.aspx?Id=guid&ScenarioId=guid [requires the MessageRest structure, returns nothing]
204 – OK, edited
412 – Transition from current message status to required status (MessageResult and MessagePhase) is not possible.
405 – MessageResult or MessagePhase are specified, but they are not consistent.
If the Forms field is filled out, then ScenarioId must be specified in the URL. If there is a form based on such a ScenarioId, it is edited, otherwise a new form is created. If there are multiple forms with the given ScenarioId, the form instance ID in the FormRest structure is considered; if no ID is specified in FormRest, the first one found according to the ScenarioId will be edited (which need not be a desirable behavior).
Culture can be entered instead of LanguageId.
ExternalKey can be entered instead of ContactId.
IssueKey can be entered instead of IssueId.
SystemName can be entered instead of AgentId.
If an agent is specified, the message is set as pre-distributed (for inbound)
RelatedMessageKey can be entered instead of RelatedMessageId.
ScenarioId is required only if forms are imported and these forms do not have any ScenarioId specified in the FormRest structure.
Creating or editing an item¶
PUT - Rest.aspx?MessageKey=MSGKEY&CampaignId=guid&ScenarioId=guid [requires the MessageRest structure, returns the item’s GUID]
200 – OK, edited
201 – OK, created
412 – Transition from current message status to required status (MessageResult and MessagePhase) is not possible.
405 – MessageResult or MessagePhase are specified, but they are not consistent. Other data are missing or are not consistent. Only when MessageResult is Active editing is possible.
If the Forms field is filled out, then ScenarioId must be specified in the URL. If there is a form based on such a ScenarioId, it is edited, otherwise or when an item is being created, a new form is created. If there are multiple forms with the given ScenarioId, the form instance ID in the FormRest structure is considered; if no ID is specified in FormRest, the first one found according to the ScenarioId will be edited (which need not be a desirable behavior).
Items cannot be created without an CampaignId in the URL (Error 405).
Culture can be entered instead of LanguageId.
ExternalKey can be entered instead of ContactId.
IssueKey can be entered instead of IssueId.
SystemName can be entered instead of AgentId.
If an agent is specified, the messgae is set/created as pre-distributed (for inbound)
RelatedMessageKey can be entered instead of RelatedMessageId.
ScenarioId is required only if forms are imported and these forms do not have any ScenarioId specified in the FormRest structure.
If MessageKey in URL is specified, than this message is searched and if not found 404 error is returned. MessageKey in MessageRest is not used for search, only as data input.
Creating an item¶
PUT - Rest.aspx&CampaignId =guid&ScenarioId=guid [requires the MessageRest structure, returns the item’s GUID]
201 – OK, created
405 – MessageResult or MessagePhase are specified, but they are not consistent. Other data are missing or are not consistent.
If the Forms field is filled out, then ScenarioId must be specified in the URL. New forms are always created.
Items cannot be created without an CampaignId in the URL (Error 405).
Culture can be entered instead of LanguageId.
ExternalKey can be entered instead of ContactId.
IssueKey can be entered instead of IssueId.
SystemName can be entered instead of AgentId.
If an agent is specified, the messgae is created as pre-distributed (for inbound)
RelatedMessageKey can be entered instead of RelatedMessageId.
ScenarioId is required only if forms are imported; this method allows the importing only of forms with the same ScenarioId.
Creating set of items¶
POST Rest.aspx?CampaignId=guid&ScenarioId=guid [requires an array of the MessageRest structures, returns an array of int items that are free of error]
Items cannot be created without an CampaignId in the URL (Error 405).
Culture can be entered instead of LanguageId.
ExternalKey can be entered instead of ContactId.
IssueKey can be entered instead of IssueId.
SystemName can be entered instead of AgentId.
If an agent is specified, the messgae is created as pre-distributed (for inbound)
RelatedMessageKey can be entered instead of RelatedMessageId.
ScenarioId is required only if forms are imported; this method allows the importing only of forms with the same ScenarioId.
Canceling an item¶
DELETE - Rest.aspx?Id=guid [cancels a record, returns nothing]
204 – OK
404 – The item does not exist.
412 – The item is not in a suitable status (MessageResult = Active).
DELETE - Rest.aspx?MessageKey=MSGKEY [returns the canceled record’s GUID]
200 – OK
404 – The item does not exist.
412 – The item is not in a suitable status (MessageResult = Active).
Sending message data¶
The PostMessage workflow node allows the MessageRest structure for a message with an InstanceRefId to be sent using POST to an external system and, if relevant, process the returned data. The MessageResultChanged and the MesagePhaseChanged triggers are called when the message state changes.
Data structures for messages¶
Data structures are described as C# classes. Items highlighted in italics are always filled out in GET operations.
MessageRest¶
Name |
Length |
Meaning |
---|---|---|
MessageType |
8 |
Type of message. Valid options are: Email, SMS, Fax, Task, Note. Optional, but if not stated, type Note is used as default. |
Direction |
1 |
Direction of message. Valid options O, I. (for Outbound or Inbound) |
SubjectField |
320 |
Subject (or Title) od a message |
BodyHtml |
2GB |
HTML formatted text of message. It will subject to XSS screening. Optional, if not supplied but BodyText yes, then BodyHtml is created as using <br> tags instead of CR/LF. |
BodyText |
2GB |
Plan text of message. Optional, if not supplied but BodyHtml yes, then BodyText is calculated by stripping all HTML tags. |
FromField |
160* |
Sender address, specific to given MessageType.
Optional Field. *Maximum length is valid for display only. |
ToFields |
Nx160* |
Array of target addresses (“TO:”). Each element is specific to given MessageType same way as FromField. Optional Field. |
ToCcFields |
Nx160* |
Array of carbon copy addresses (“CC:”). Used only for MessageType=Email. |
ToBccFields |
Nx160* |
Array of blind carbon copy addresses (“BCC:”). Used only for MessageType=Email. |
ScheduleTime |
This is the time for which the outbound message is scheduled, expressed in local time. The message will not be sent before until this time. JSON format: “2016-06-27T19:15:01.9706909+02:00”. |
|
Skill |
This is a value from 0 to 100 that specifies a minimum skill of the agent to whom the message will be distributed. Messages are imported to the project specified for the given campaign (CampaignId). If no skill is explicitly stated, then the skill specified for the given campaign is used (CampaignId). For outbound messages of type Email, SMS, Fax and Note it has no impact since they are not distributed. |
|
LanguageId |
This is an internal key (GUID) of the language in which the message is to be managed. If it contains “00000000-0000-0000-0000-000000000000”, the field is deleted. If it is specified, then it must be one of the keys in the Languages table, otherwise the message is not inserted/changed. |
|
Culture |
This is a two-letter ISO code of the language in which the message is to be managed. If it is specified, it must be one of the keys in the Languages table, otherwise the message is not inserted/changed. If both Culture and LanguageId are stated, then Culture is used. If no language is explicitly stated, then the language specified for the given campaign is used (CampignId), provided that it is at all specified. |
|
Proficiency |
This is a value from 0 to 100 that specifies a minimum language proficiency of the agent to whom the message will be distributed. If no proficiency is explicitly stated, then the proficiency specified for the given outbound campaign is used (CampignId), provided that it is at all specified. For outbound messages of type Email, SMS, Fax and Note it has no impact since they are not distributed. |
|
Priority |
This is a value from 0 to 100 that specifies the message priority (with 100 being the highest); messages with a higher priority are distributed earlier. If no priority is explicitly stated, then the priority specified for the given outbound campaign is used (CampignId), provided that it is at all specified. For outbound messages of type Email, SMS, Fax and Note it has no impact since they are not distributed. |
|
Mark |
This is an application mark; an integer from -2147483647 to 2147483647. |
|
ContactId |
This is an internal key (GUID) of the contact with which the message is to be associated. If it contains “00000000-0000-0000-0000-000000000000”, the Contact field is deleted. If the key does not exist or is NULL, the data is not changed. If this key is not found in the Contact table, then Error 400 occurs. |
|
ExternalKey |
48 |
This is an optional external key of the contact that with which the message is to be associated. If both ContactId and ExternalKey are specified, then ExternalKey is used. If it contains an empty string “”, the Contact field is deleted. If the key does not exist or is NULL, the data is not changed. If this key is not found in the Contact table, then Error 400 occurs. |
AgentId |
This is an internal key (GUID) of the agent to whom the message is to be assigned (using the pre-distribution mechanism). If it contains “00000000-0000-0000-0000-000000000000”, the Agent field is deleted. If the key does not exist or is NULL, the data is not changed. If this key is not found in the AgentId table, then Error 400 occurs. |
|
SystemName |
200 |
This is a system login name of the agent to whom the message is to be assigned (using the pre-distribution mechanism). If both AgentId and SystemName are specified, then SystemName is used. If it contains an empty string “”, the Agent field is deleted. If it does not exist or is NULL, the data is not changed. If the key is not found in the Agent table, Error 400 occurs. |
SpamLevel |
This is number of spam level, if it has been assigned. missing/null – not assigned 0 – no spam 1 – manually detected spam 2 – automatically detected spam in FS 3 – externally detected spam |
|
SignatureValidity |
Digital signature validity for an Email. |
|
IssueId |
This is an internal key (GUID) of the issue with which the message is to be associated. If it contains “00000000-0000-0000-0000-000000000000”, the Issue field is deleted. If the key does not exist or is NULL, the data is not changed. If this key is not found in the Issue table, then Error 400 occurs. |
|
IssueKey |
48 |
This is an optional external key of the issue with which the message is to be associated. If both IssueId and IssueKey are specified, then IssueKey is used. If it contains an empty string “”, the Issue field is deleted. If the key does not exist or is NULL, the data is not changed. If this key is not found in the Issue table, then Error 400 occurs. |
MessageKey |
48 |
This is an optional external key of the message, which is used for synchronization with external data sources. The key uniqueness is not checked during batch processing. |
Forms |
This is an array of FormRest structures with data from the associated data forms. The form scheme is determined by the ScenarioId parameter in the URL with the following effects:
|
|
Attachments |
This is an array of AttachmentRest structures with data of the associated attachments. The binary part (BinaryContent64 field) is filled in GET operations only when WithBinary is specified in URL. Attachment binary content can also by read by specific API as normal GET/POST download/upload. |
|
Id |
This is an internal key (GUID) of the message. It is specified for the GET operations, otherwise it is ignored. |
|
RemoteAddress |
250 |
This is purged address of remote correspondent, irrespective on direction. For Email it is email address, for Fax and SMS it is a phone number. |
MessageResult |
16 |
This is the message result (status). It can contain the following values:
It is filled out during GET operations. When making changes in PUT/POST, it is allowed to specify it only for new messages or for messages in Active status. |
MessagePhase |
16 |
This is the processing phase. It can contain the following values for MessageTypes Email, SMS, Fax – inbound direction:
For MessageTypes Email, SMS, Fax – outbound direction:
For MessageType Task:
For MessageType Note:
This is filled out during GET operations. When making changes in PUT/POST, it is allowed to specify it only for new messages (phases marked by *) or for messages in Active status (phases marked by †) |
RelatedMessageId |
This is an internal key (GUID) of the existing messsage with which the message is to be associated. If it contains “00000000-0000-0000-0000-000000000000”, the RelatedMessage field is deleted. If the key does not exist or is NULL, the data is not changed. If this key is not found in the Message table, then Error 400 occurs. |
|
RelatedMessageKey |
48 |
This is an external key of the message with which the message is to be associated. If both RelatedMessageId and RelatedMessageKey are specified, then RelatedMessageKey is used. If it contains an empty string “”, the RelatedMessage field is deleted. If the key does not exist or is NULL, the data is not changed. If this key is not found in the Issue table, then Error 400 occurs. |
DraftTime |
This is the time of creating outbound message. This is filled out during GET operations, can be specified for POST/PUT of messages. If not specified for Active message or Task, it is set to current local time. |
|
ReceivedSentTime |
This is the processing start time. It only applies to messages that has been received or sent (depending on direction). This is filled out during GET operations, can be specified for POST/PUT of messages. |
|
ReadConfirmedTime |
This is the time when message when it has been read or confirmed (depending on direction). This is filled out during GET operations, can be specified for POST/PUT of messages. |
|
AnsweringTime |
This is the message answer time. It only applies to messages that reached the phase Answering. This is filled out during GET operations, it can be specified for POST/PUT of not active messages. |
|
EndTime |
This is the message processing end time. It only applies to completed or terminated messages (not Active). This is filled out during GET operations, should be specified for POST/PUT for not active messages. |
|
ProjectId |
ProjectID (GUID) of given message. Filled during GET. |
|
CampaignId |
CampaignID (GUID) of given message. Filled during GET. |
|
GatewayId |
GatewayID (GUID) of given message. Filled during GET. It can be specified for active outbound messages of type Email, SMS, Fax in POST/PUT operations in phase Draft or Scheduled. If specified, it overrides MessageScheduleCondition rules. |
public class MessageRest
{
public string MessageType { get; set; }
public string Direction { get; set; }
public string SubjectField { get; set; }
public string BodyHtml { get; set; }
public string BodyText { get; set; }
// simple syntax: "some@example.com" or extended sytax: "Display Name<some@example.com>"
public string FromField { get; set; }
public string[] ToFields { get; set; }
public string[] ToCcFields { get; set; }
public string[] ToBccFields { get; set; }
public DateTime? ScheduledTime { get; set; }
public int? Skill { get; set; }
public Guid? LanguageId { get; set; }
public string Culture { get; set; } // alternative to LanguageId
public int? Proficiency { get; set; }
public int? Priority { get; set; }
public int? Mark { get; set; }
public Guid? ContactId { get; set; }
public string ExternalKey { get; set; } // alternative to ContactId
public Guid? AgentId { get; set; }
public string SystemName { get; set; } // alternative to AgentId
public int? SpamLevel { get; set; }
public int? SignatureValidity { get; set; }
public FormRest[] Forms { get; set; }
public AttachmentRest[] Attachments { get; set; }
public string MessageKey { get; set; }
public Guid? Id { get; set; }
public string MessageResult { get; set; } // write is subject to high permission
public string MessagePhase { get; set; }
public Guid? RelatedMessageId { get; set; }
public string RelatedMessageKey { get; set; } // alternative to RelatedMessageId
public DateTime? DraftTime { get; set; }
public DateTime? ReceivedSentTime { get; set; }
public DateTime? ReadConfirmedTime { get; set; }
public DateTime? AnsweringTime { get; set; }
public DateTime? EndTime { get; set; }
public Guid? ProjectId { get; set; }
public Guid? CampaignId { get; set; }
public Guid? GatewayId { get; set; }
public Guid? IssueId { get; set; }
public string IssueKey { get; set; }
}
Data example¶
MessageRest data structure:
{
"MessageType": "Note",
"Direction": "O",
"SubjectField": "Obrázek2.png",
"BodyHtml": "<i>Obrázek2.png</i>",
"BodyText": "Obrázek2.png",
"Priority": 70,
"Mark": 0,
"ContactId": "c9f6103d-b57c-4c69-91b8-0e5d6e2f24b0",
"Forms": [],
"Attachments": [
{
"DisplayName": "Obrázek2.png",
"Mode": "Normal",
"FileName": "Obrázek2.png",
"MediaType": "image/png",
"PartIndex": 0,
"ContentSize": 56759,
"BinaryContent64": "iVBORw0KGgoAAAANSUhEUgAAB.........AIE6AAAAAAAsQKAOAAAAAAALEKgDAAAAAMACBOoAAAAAALAAgToAAAAAAHxRxP8Pqccd3z+R8jQAAAAASUVORK5CYII=",
"Id": "abd9b0ff-de10-eb11-8afe-bce92ff829d5",
"MessageId": "a5d9b0ff-de10-eb11-8afe-bce92ff829d5"
}
],
"Id": "a5d9b0ff-de10-eb11-8afe-bce92ff829d5",
"MessageResult": "Closed",
"MessagePhase": "Closed",
"DraftTime": "2020-10-18T03:12:33.267",
"ReceivedSentTime": "2020-10-18T03:12:33.267",
"EndTime": "2020-10-18T03:12:33.267",
"IssueId": "ef454e37-05f2-e711-8bd5-0028f8303aaa"
}
{
"MessageType": "Email",
"Direction": "O",
"SubjectField": "RE: Test nezávisle 1",
"BodyHtml": "<p>Skupina <strong>atlantis</strong> se specializuje na oblast hlasových a datových komunikačních technologií a vývoj softwaru. Jako na producentech nezávislý dodavatel komunikačních řešení můžeme pro své zákazníky vybírat ty nejlepší produkty, které se na celosvětovém trhu objeví.</p><br>",
"BodyText": "",
"FromField": "FS-Volný <frontstage@volny.cz>",
"ToFields": [
"zidek@seznam.cz"
],
"ScheduledTime": "2018-08-11T02:50:00",
"Skill": 10,
"Priority": 70,
"Mark": 0,
"AgentId": "4354b36d-e2ab-46ad-bc78-347edcd9b293",
"SystemName": "atlantis2000\\\\zidek",
"Forms": [],
"Attachments": [],
"MessageKey": "M111",
"Id": "6b0283bc-ff9c-e811-8c07-40b034090009",
"MessageResult": "Active",
"MessagePhase": "Scheduled",
"RelatedMessageId": "d841310b-e469-e811-8bf7-40b034090009",
"ReadConfirmedTime": "2019-03-08T16:12:31",
"ProjectId": "18f26f7a-0c97-4a66-89a7-7299253c0a99",
"CampaignId": "97c4380a-6bb3-496e-a8a0-c13172e73b0e",
"IssueId": "727c9213-e469-e811-8bf7-40b034090009"
}
Attachments¶
Attachments are uniquely identified only using their Id. Attachments cannot exist in FS without their parent Message record. However, there is simplified way to add an attachment and automatically create related parent note. This is specifically designed for adding attachments to Issues or Contatcs.
Processing attachment data¶
Attachment REST operations are specific, because they do not use JSON payload. For PUT operation the payload is directly the content and HTTP header ContentType is honored as well as other headers, file name can be supplied in URL as parameter Name (encoded). For POST operation the ContentType has to be of type “multipart/form-data”, and allows sending multiple files; each part properties like ContenType or FileName are honored. POST or PUT operations create always attachments with mode “Normal”. When creating attachments to Issue or Contact optional title of newly created Note can by specified by parameter Title (encoded).
The required authorization is EditMessage, for GET it is AllowRead, and for PUT (replacement)/DELETE it is AllowFull. POST/PUT operations require only AllowWrite for newly created messages of type Note, or for messages in these states (error 412 would be returned).
MessageType / Direction |
MessageResult |
MessagePhase |
---|---|---|
Email / O |
Active |
Draft |
Fax / O |
Active |
Draft |
Note / O,I |
Active |
Draft |
Task / O,I |
Active |
New, Read, Open, Hold, Wait |
Otherwise AllowFull is required and no limitation is imposed. Editors do not show attachments for some MessageType.
Downloading item¶
GET - Rest.aspx?Id=guid [returns the binary content of type Attachment. ContentType, ContentDisposition and other attributes of download are set according to AttachmentRest]
200 – OK
404 – attachment with given Id not found
Replacing existing item¶
PUT - Rest.aspx? Id=guid [returns the AttachmentRest structure of created attachment with filled Id and related MessageId and MessageKey]
200 – OK
404 – attachment not found
412 – attachment with given Id is of different mode than Normal
Uploading new item for message¶
PUT - Rest.aspx?MessageId=guid OR MessageKey=MSGKEY & Name=Name [returns the AttachmentRest structure of created attachment with filled Id and related MessageId and MessageKey]
200 – OK
404 – message not found
Uploading new item for issue¶
PUT - Rest.aspx?IssueId=guid OR IssueKey=ISSUEKEY & Name=Name & Title=Title [returns the AttachmentRest structure of created attachment with filled Id, and created Note MessageId]
200 – OK
404 – issue not found
Uploading new item for contact¶
PUT - Rest.aspx?ContactId=guid OR ExternalKey=EXTERNALKEY & Name=Name & Title=Title [returns the AttachmentRest structure of created attachment with filled Id, and created Note MessageId]
200 – OK
404 – contact not found
Uploading new items for message¶
POST - Rest.aspx?MessageId=guid OR MessageKey=MSGKEY [returns the array of AttachmentRest structures of created attachments with filled Id and related MessageId and MessageKey]
200 – OK
404 – message not found
Uploading new items for issue¶
POST - Rest.aspx?IssueId=guid OR IssueKey=ISSUEKEY & Title=Title [returns the array of AttachmentRest structures of created attachments with filled Id and related MessageId and MessageKey]
200 – OK
404 – issue not found
Uploading new items for contact¶
POST - Rest.aspx?ContactId=guid OR ExternalKey=EXTERNALKEY & Title=Title [returns the array of AttachmentRest structures of created attachments with filled Id and related MessageId and MessageKey]
200 – OK
404 – contact not found
Deleting an item¶
DELETE - Rest.aspx?Id=guid [deletes attachment of GUID, but only when it is of mode Normal]
204 – OK
404 – attachment with given Id not found
412 – attachment with given Id is of different mode than Normal
Data structures for attachments¶
Data structures are described as C# classes. Items highlighted in italics are always filled out in GET operations.
AttachmentRest¶
Name |
Length |
Meaning |
---|---|---|
DisplayName |
320 |
Name of the attachment. Used only when part of MessageRest structure. |
Mode |
32 |
Type of attachment. For GET operation these are possible:
For POST/PUT operations only these are allowed:
Used only when part of MessageRest structure. |
FileName |
1024 |
File name used for download. This is expected to be valid file name. Used only when part of MessageRest structure. |
MediaType |
320 |
MIME type specification of content
Example: text/plain Used only when part of MessageRest structure. |
PartIndex |
Order number used for presentation and for converting to EML. If omitted for POST/PUT, automatically calculated. It starts in 0. Used only when part of MessageRest structure. |
|
ContentId |
128 |
Identifier of the content within EML represenantion that can be used for referencing in tags (like <img href=”xxxxx” />) within BodyHtml. Relevant only for Resource and EmlResource mode of attachment. Example: image001.png@01CEC597.1BB93820 Used only when part of MessageRest structure. |
SignatureValidity |
Digital signature validity for an attachment. Relevant only for Normal or EmlAttachement modes. Used only when part of MessageRest structure. |
|
ContentSize |
Size of attachment in bytes, if known. Typically it is known for Normal and Resource attachment modes. Used only when part of MessageRest structure. |
|
Id |
This is an internal key (GUID) of the attachment. It is specified for the GET operations only when part of MessageRest structure. And in responses to POST operations (for binary attachments), otherwise it is ignored. |
|
MessageId |
Internal key (GUID) of the message, to which the attachment is related (parent ID). It is specified in responses to POST operations (for binary attachments), otherwise it is ignored. |
|
MessageKey |
48 |
This is an optional external key of the message, which is used for synchronization with external data sources. It is specified in responses to POST operations (for binary attachments), otherwise it is ignored. |
BinaryContent64 |
2GB |
Used only when part of MessageRest structure and filled in GET operations only when WithBinary is specified in URL. Attachment binary content can also be read by specific API as normal GET/POST download/upload. |
public class AttachmentRest
{
public string DisplayName { get; set; }
public string Mode { get; set; }
public string FileName { get; set; }
public string MediaType { get; set; }
public int? PartIndex { get; set; }
public string ContentId { get; set; }
public int? ContentSize { get; set; }
public string BinaryContent64 { get; set; }
public int? SignatureValidity { get; set; }
public Guid? Id { get; set; }
public Guid? MessageId { get; set; }
public string MessageKey { get; set; }
}
Data example¶
Invoked URL with PUT, creating new item:
http://*server/WebAdmin*/Pages/Attachments/Rest.aspx?MessageId=43220169-6dbe-e911-8c74-40b034090009&Name=Obr%u00e1zek2.png
{
"DisplayName": "Obrázek2.png",
"Mode": "Normal",
"FileName": "Obrázek2.png",
"MediaType": "image/png",
"PartIndex": 4,
"ContentSize": 56759,
"Id": "acbf6553-de10-eb11-8afe-bce92ff829d5",
"MessageId": "43220169-6dbe-e911-8c74-40b034090009"
}
Invoked URL with POST, creating new item:
http://*server/WebAdmin*/Pages/Attachments/Rest.aspx?IssueId=fd5344f7-3704-eb11-8aef-ccd9ac50fd24&Name=Obr%u00e1zek2.png&Title=Ob%u010dansk%u00fd+pr%u016fkaz
[
{
"DisplayName": "Diagram_iCCV315 po změnách.png",
"Mode": "Normal",
"FileName": "Diagram_iCCV315 po změnách.png",
"MediaType": "image/png",
"PartIndex": 0,
"ContentSize": 61712,
"Id": "288b80a0-5d11-eb11-8b00-bce92ff829d5",
"MessageId": "4a86af8d-5d11-eb11-8b00-bce92ff829d5"
}
]
Result in RC editor:
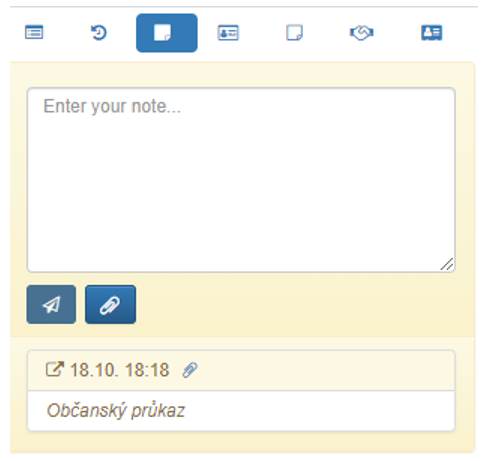
Forms¶
Forms are uniquely identified by their Id.
Processing message data¶
During REST operations with forms (ScenarioResult, Form), both direct and indirect ones, when the form is a part of a superior structure, the relevant synchronous sections of OnCreateSqlCmd and OnSaveSqlCmd are called, as defined in the scenario header, with the context (@Context) being equal to “REST”, yet the FormSaved triggers not being called. If the synchronous SQL part of such a trigger should be executed, we recommend including it in OnSaveSqlCmd; if the asynchronous part should be executed, we recommend calling it manually by creating a record in the WorkflowInstance table.
The required authorization is EditForm, for GET it is AllowRead, and for PUT/POST/DELETE it is AllowWrite.
Reading an item¶
GET - Rest.aspx?Id=guid [returns the FormRest structure]
Reading an item array¶
GET - Rest.aspx?Skip=10&Take=20&ScenarioId=guid&Completed=true&Valid=true [returns the FormRest array]
ScenarioId is always obligatory, only Forms according to this scenario definitions are returned up to max. 1000 items.
If Completed=true is specified, then only forms with Activity=Completed are returned.
If Valid=true is specified, then only forms with Activity=Completed or Activity=Active are returned.
Editing an item¶
PUT - Rest.aspx?Id=guid [requires the FormRest structure, returns nothing]
204 – OK, edited; if Activity of form was PreFilled then it changes to Active automatically
412 – The form is in Completed or Cancelled state and cannot be changed. When user has right to EditForm as Full, then editing of Completed forms is possible.
Creating an item¶
PUT - Rest.aspx&ScenarioId=guid&Prefilled=true [requires the FormRest structure, returns the item’s GUID]
201 – OK, created
ScenarioId is required (otherwise Error 405)
If PreFilled=true is specified, the new form Activity will remain as PreFilled
Creating set of items¶
POST Rest.aspx?ScenarioId=guid&Prefilled=true [requires an array of the FormRest structures, returns an array of int items that are free of error]
Items cannot be created without an ScenarioId in the URL (Error 405); this method allows the importing only of forms with the same ScenarioId.
If PreFilled=true is specified, the new form Activity will remain as PreFilled
Canceling an item¶
DELETE - Rest.aspx?Id=guid [cancels a record, returns nothing]
204 – OK
404 – The item does not exist.
412 – The item is not in a suitable status (Activity = Active or PreFilled).
Sending form data¶
The PostForm workflow node allows the FormRest structure for a form with an InstanceRefId to be sent using POST to an external system and, if relevant, process the returned data. The FormSaved triggers is called when the form data changes.
Data structures for Form¶
Data structures are described as C# classes. Items highlighted with italics are always filled out in GET operations.
Name |
Length |
Meaning |
---|---|---|
Data |
These are name-value pairs for the form data field. The field names are defined by the form scheme. A name can have up to 32 characters. The value is interpreted in accordance with the field type in the scheme. Possible field types and their interpretation:
|
|
ScenarioId |
An internal ID (GUID) of the form scheme. |
|
Id |
An internal key (GUID) of the form. |
FormRest¶
public class FormRest
{
public Dictionary<string,string> Data { get; set; }
* public Guid? ScenarioId { get; set; }*
* public Guid? Id { get; set; }*
}
Example of data¶
FormRest structure:
{
"Data": {
"Subject": "SaleAA",
"A4": null,
"MemberNumber": "9999",
"PreferredContact": null,
"BaggageNo": "11588",
"Color": null,
"Remark": "",
"Delivery": null,
"Solution": "refund;exchange",
"Remarks": "New remark !"
}
}
Entities¶
Entities allow only data retrieval of multiple items
Processing entities data¶
This REST operation allows sending any mix of Contacts, Issues, Messages, OutboundCalls and Forms using data structures defined in this document. Selection of entities is determined by executing custom DataQuery which is supplied an parameter @RecordId. The DataQuery has to return two colums: Id – Guid of entity and EntityType – name of the entity (literal text – one of list “Contact”, “Issue”, “Message”, “OutboundCall” or “Form”).
The required right is AllowRead for respective entities and for DataQuery right.
Reading an item array¶
GET - Rest.aspx?Id=guid&DataQueryId=guid&WithBinary=true&WithForms=true [returns the EntityRest field]
Id is required parameter, it is passed to DQ as @RecordId variable.
DataQueryId is required parameter, it must reference DQ. Besides @RecordId the DQ receives filled also other variables like @MeAgentId
Returns maximum of SnapshotsMaxRecords items at once (defaults to 2000)
If WithBinary=true is specified, then fields of BinaryContent64 are filled, otherwise only metadata of attachments are supplied.
If WithForms=true is specified, then associated forms for entities OutboundCall and Message are filled with Data, otherwise only Ids and Scenarios are filled in their FormRest structure. When entity of type Form is to be exported by result from DQ, it always contains Data. It concerns only Related forms. Primary form of Issue and Contact are always filled.
Sending entities data¶
The PostEntities workflow node allows the array of EntityRest structure InstanceRefId (@RecordId) to be sent using POST to an external system and, if relevant, process the returned data.
Data structures for Form¶
Data structures are described as C# classes. Items highlighted with italics are always filled out in GET operations.
Name |
Length |
Meaning |
---|---|---|
Id |
GUID of exported entity (copied from source DataQuery) |
|
EntityType |
32 |
Name of exported entity (copied from source DataQuery). Possible values are:
|
Contact |
Exported contact in ContactRest structure. Only if EntityType is Contact. Associated forms exported only if WithForms=true. Main form exported always. |
|
Issue |
Exported issue in IssueRest structure. Only if EntityType is Issue. Associated forms exported only if WithForms=true. Main form exported always. |
|
OutboundCall |
Exported outbound call in OutboundCallRest structure. Only if EntityType is OutboundCall. Associated forms exported only if WithForms=true. |
|
Message |
Exported message in Message Rest structure. Only if EntityType is Message. Associated forms exported only if WithForms=true. If |
|
Form |
Exported form in FormRest structure. |
FormRest¶
public class EntityRest
{
public Guid Id { get; set; }
public string EntityType { get; set; } // Contact, Issue, OutboundCall, Message, Form
public ContactRest Contact { get; set; }
public IssueRest Issue { get; set; }
public OutboundCallRest OutboundCall { get; set; }
public MessageRest Message { get; set; }
public FormRest Form { get; set; }
}
Example of data¶
EntitiyRest structure:
DataQuery¶
DataQuery allows only data retrieval of general query as rows.
Processing rows data¶
This REST operation allows sending arbitrary data defined by custom DataQuery. Selection of data is determined by executing custom DataQuery which is supplied a parameter @RecordId. Optionally it is possible to add in URL column filters. Sort order is done according to DataQuery default sorting settings. Returned colums are all defined DataQueryColums except for models Css, Color, Bold, QueryText, QueryId, Command and Toggle. Data are formatted as JSON retaining their data type. No formatting or conversion is performed, with exception of DateTimeUtc model, which is converted to server local time. Data are returned as array of JSON objects, these objects has properties named according to DataQueryColum DisplayName.
The required right is AllowRead for DataQuery.
Note: No XSS sanitization is performed on output data.
Reading an item array¶
GET - Rest.aspx?Id=guid&DataQueryId=guid&Skip=000&Take=999&Sort=colname&FilterColName1=value&… [returns the array of objects]
Id is optional parameter, it is passed to DQ as @RecordId variable.
DataQueryId is required parameter, it must reference DQ. Besides @RecordId the DQ receives filled also other variables like @MeAgentId
Skip is optional parameter, that allows to tell how many records should be skipped (from start)
Take is optional parameter, that allows to tell how many records be returned
Returns maximum of SnapshotsMaxRecords items at once (defaults to 2000)
Sort is optional parameter that can specify which DataQueryColumn should be used as sorting (if the default sorting is not desired), name should match DisplayName
Reverse is optional parameter that can reverse sorting direction (value “true”)
All other parameters are tested against DataQueryColum DisplayName and if match, they are considered to be filter text values (similar to values that user can enter on web pages with Data Query Grids).
Example
http://localhost:60600/WebAdmin/Pages/DataQueries/Rest.aspx?DataQueryId=ded5e653-9122-4a62-9e59-461da3f5d643&Číslo=0602*&Stav=Lost&Skip=10&Take=5&Sort=Čas&Reverse=true
[
{
"Stav": "Lost",
"Fáze": "AgentRingFirst",
"Čas": "2020-07-22T00:24:34.587",
"Číslo": "0602330533",
"Značka": 0,
"Agent": "Jakub Sadil",
"Preferovaný": null,
"Projekt": "Infolinka",
"Jazyk": null,
"Pracoviště": "W161",
"Pilot": "P168",
"Trvání": null,
"Výsledek": null,
"IVR A": null,
"Pokrač.": null,
"Zvonění": 14,
"Vstup": "",
"Kontakt": "Pablo Neruda "
},
{
"Stav": "Lost",
"Fáze": "WaitingQueue",
"Čas": "2020-06-24T18:30:24.723",
"Číslo": "0602330533",
"Značka": 0,
"Agent": null,
"Preferovaný": null,
"Projekt": "Infolinka",
"Jazyk": "Angličtina",
"Pracoviště": null,
"Pilot": "P168",
"Trvání": null,
"Výsledek": null,
"IVR A": "",
"Pokrač.": null,
"Zvonění": null,
"Vstup": "521",
"Kontakt": "Pablo Neruda "
}
]
Sending rows data¶
The PostDataQuery workflow node allows the array of JSON objects. InstanceRefId (@RecordId) to be sent using POST to an external system and, if relevant, process the returned data.
Raw data¶
Receiving raw data¶
A special page is used to transfer raw data to FrontStage, specifically to the ChangeRequest table.
The required authorization is RestRpc AllowWrite for GET/PUT/POST/DELETE.
Data from the ChangeRequest table can be processed using SQL, e.g. by an interval trigger.
Creating an item¶
An item can be created using any of the following http methods: POST/PUT/GET/DELETE. [The returned value is always the GUID of the created record in the ChangeRequest table].
METHOD AcceptData.aspx&Number=int&ReferenceId=guid&SubjectId=guid&DataId=guid&Text=AB&TimeUtc=datetime
METHOD – This is recorded in the Command column as one of these columns:
AcceptDataPost
AcceptDataPut
AcceptDataGet
AcceptDataDelete
Number – An integer that is recorded in the Number column
TimeUtc – A time in the JSON format (e.g. 2012-03-19T07:22Z)
ReferenceId – A GUID that is recorded in the ReferenceId column
SubjectId – A GUID that is recorded in the SubjectId column
DataId – A GUID that is recorded in the DataId column
Text – This is considered just for GET and DELETE; it is recorded in the Text column.
For POST and PUT, the http request body is copied to the Text column as is.
All parameters are optional.
If the writing is successful, the system returns 200 OK, otherwise Error 401 or 422 depending on the problem type.
Other fields are automatically filled out as follows:
ChangeRequestId – a sequence GUID
ChangeRequestTimeUtc – a record creation time in UTC
ActorId – an ID of the authenticated agent who makes the record
Result – NULL, not filled out
Done – 0 (false)
InProgress – 0 (false)
ChangeRequest table structure¶
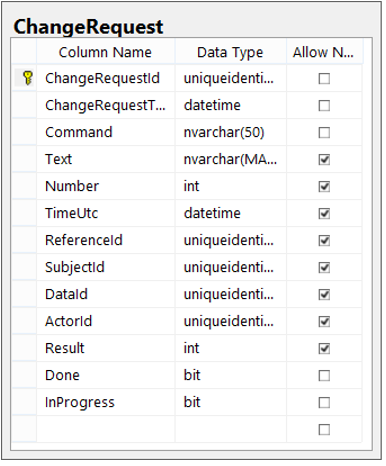
Sending raw data¶
The WF QueryUrlText, QueryUrlCsv and QueryUrlJson nodes make it possible to send data in the URL and process the returned data in the format of a single field (Text), single CSV row or a glossary structure (Json) – analogously to the Data field from FormRest.