WebSite module integration¶
The WebSite module offers rich options for integrating embedded applications with the contact center operator’s website. Integration is possible through three main methods, offering different levels of adaptability and complexity. The easiest is embedding with JavaScript, but more options are offered by JSON/HTTP AP. The most demanding but fully customizable integration is achieved using the SimProt/TCP protocol.
Integration options¶
The integration into the website is performed by the website implementer, i.e. the one who is able to change the code or configure the main web server. The integration is possible on three levels:
Integration by inserting a link to JavaScript - It renders the chat into an HTML page and ensures data communication with the WebSite module.
Integration at the level of data communication - the JSON format via http(s); the visual representation is fully under the control of the web presentation author.
Integration at the level of data communication with the FrontStage server - .NET4 + compatible DLL is available, or SimProt messages transmitted by TCP/IP are used. In this case, WebSite is not used at all.
Integration with common IM networks is assumed using the prepared App.ServiceLink connector gateway and is performed by the account manager in these networks (e.g. Facebook account, Twitter account, Viber account, etc.); however, this integration is generally reduced to access data settings (ID , password, etc.). Integration with any other IM platform is possible via a custom-programmed connector.
The following table summarizes the options for level online presentation integration:
Integration level |
WebSite: JavaScript |
WebSite: JSON/http(s) |
Chat: SimProt/TCP |
---|---|---|---|
Integration point |
HTML |
Client browser |
Server |
Change of appearance |
Minor, at the CSS editing level |
Major, fully under control |
Major, fully under control |
Change of behavior/logic |
None except for configuration |
Medium, just logic on the client side |
Major, a possibility to integrate with the server and the client logic |
Deployment speed |
Very fast, just inserting a link to the script into the pages |
Medium some client-side development required (HTML/JS) |
Slow, requiring considerable development on the server side of the web (PHP, Java, ASP.NET,…) |
Client-side browser compatibility |
Provided by iCC.WebSite, i.e. atlantis as part of upgrades |
The browser must support JavaScript; adaptation for different browsers is provided by the implementer. |
Completely provided by the implementer; all kinds of technical solution possibilities. |
The following table summarizes the options for levelsof instant messaging network integration:
Integration level |
WebSite: JSON/http(s) |
Chat: SimProt/DLL |
Chat: SimProt/TCP |
---|---|---|---|
Integration point |
HTTP REST API iCC.WebSite |
.NET 4 Assembly (C# VB.NET F#) |
TCP (formatted packets) |
Transmission speed |
Medium, new messages detected by polling |
High, instant message delivery |
High, instant message delivery |
Deployment speed |
Medium Some development using WebAPI required (REST+JSON) |
Medium, some development using .NET tools required |
Slow, considerable development required, but any platform can be used (Java, PHP …) |
WebSite integration using JavaScript¶
The easiest way to integrate is to embed JavaScript in the contact center operator’s website (usually in the <head>
section).
Applications that are rendered in a visible form use the template (.html
extension) and settings (.json
extension) files located in the Content
folder for their rendering. The default templates have the same name as the application name. A standard script template that has the .js
extension is used depending on the type of application.
See also
Continue with the description of JavaScript integration by application:
WebSite integration using REST API¶
This integration assumes the use of the REST API (JSON/HTTP) provided by the WebSite module. WebSite itself uses the SimProt/DLL interface and acts as an external chat application.
See also
Here you will find common information. Proceed as follows to describe the integration using the REST API according to the application:
Communication between the browser and the WebChat server¶
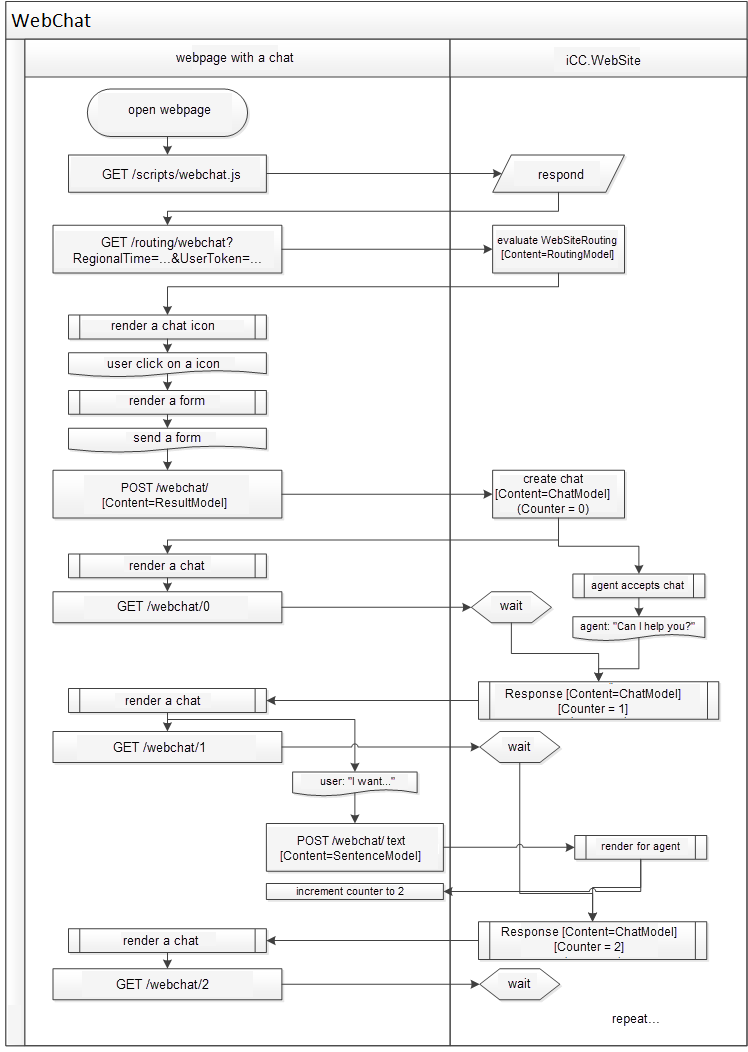
This API page concerns the website visitor. The following functions are available in the API. Deuring each call, a TrackingCookie is set that contains the visitor’s TrackingId. The user always has one chat thread available, no matter how many browser windows they have open.
Important
As a result, the conversation thread does not identify with the visitor as much as with the browser. For example, if a visitor uses two different browsers on the same computer, they will act as two independent people with two different conversations to the application.
Routing¶
The routing function should be called by the page script first right after the page is loaded to determine if and how the application is configured for the page. If for a given application
[GET] ~/api/Routing/webapp?RegionalTime=nnnnn&UserToken=tttttt&IncludeSettings=true - returns the RoutingModel structure based on information from the page and the application type. The parameters in the URL have the following meaning:
RegionalTime - Time in the form of a number as returned by the JavaScript function
Math.floor (Date.now ())
; it serves both to distinguish http requests and to prevent caching of this information in the browser and intermediate proxies, and can be used to detect the visitor’s time zone and indicate the same to the agent.UserToken - A free string of up to 320 characters designed to distinguish server-side requests; it can be used, for example, to indicate whether a visitor is logged in or not, etc. It can be evaluated by the WebSiteRouting conditions as well as by ChatGateCondition.
IncludeSettings - The parameter can be set to “true” or “false” and indicates that the server should also complete the additional fields of HtmlTemplate (a configured HTML template), Settings (configured parameters), Literals (a set of localized texts) - primarily intended for use for a out-of-the-box JavaScript integration.
The answer to this request is, depending on the application type, the RoutingModel JSON structure, where the relevant data is filled in. If NULL
is returned, then the application is not configured for the page and input parameters or is blocked and should not be rendered.
AgentImage¶
[GET] ~/api/AgentImage/agentid?width=000&height=000 - Returns an image representing the agent with a given agentid GUID; optionally, you can specify the height and width of the generated image to which the image is rescaled; the system returns only the image of the agent associated with the given TrackingCookie; the agent is used in the URL only for the possibility of caching on the browser side; if the agent does not match the agent involved in the chat, a generic image will be returned.
Data structures¶
Data structures are described below as a C# class. Serialization is performed in JSON; PascalCasing of members is preserved. The GUID type is serialized as a string.
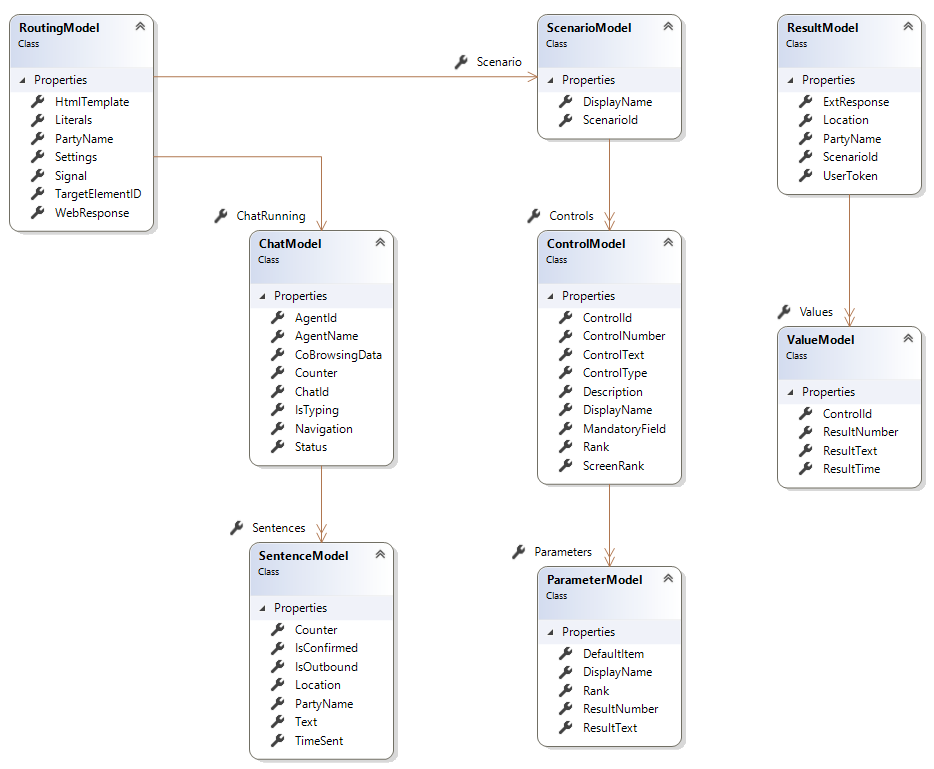
RoutingModel¶
public class RoutingModel
{
public string TargetElementID { get; set; }
public string WebResponse { get; set; }
public ScenarioModel Scenario { get; set; }
public string Signal { get; set; } // Free, Busy, Closed, Hidden
public ChatModel ChatRunning { get; set; }
public string HtmlTemplate { get; set; }
public Dictionary<string, string> Settings { get; set; }
public Dictionary<string, string> Literals { get; set; }
public string PartyName { get; set; }
public int? SolicitationTimeOut { get; set; }
public int? SolicitationDuration { get; set; }
public Guid WebSiteRoutingId { get; set; }
}
ScenarioModel¶
public class ScenarioModel
{
public Guid ScenarioId { get; set; }
public string DisplayName { get; set; }
public string Culture { get; set; }
public List<ControlModel> Controls { get; set; }
}
ControlModel¶
public class ControlModel
{
public Guid ControlId { get; set; }
public int ScreenRank { get; set; }
public int Rank { get; set; }
public string DisplayName { get; set; }
public string Description { get; set; }
// Same constants as a ScreenControl_ControlType.* but limited selection
public string ControlType { get; set; } // LabelOnly, TextArea, DropDown, RadioButtons, CheckBoxes, Integer, Date, Time, DateTime
public int? ControlNumber { get; set; }
public string ControlText { get; set; }
public bool MandatoryField { get; set; }
public List<ParameterModel> Parameters { get; set; }
}
ParameterModel¶
public class ParameterModel
{
public int Rank { get; set; }
public string DisplayName { get; set; }
public int ResultNumber { get; set; }
public string ResultText { get; set; }
public bool DefaultItem { get; set; }
}
ResultModel¶
public class ResultModel
{
public Guid? ScenarioId { get; set; }
public string Culture { get; set; }
public List<ValueModel> Values { get; set; }
public string UserToken { get; set; }
public string ExtResponse { get; set; }
public string PartyName { get; set; }
public string Location { get; set; }
public Guid WebSiteRoutingId { get; set; }
}
ResultModel¶
public class ResultModel
{
public Guid? ScenarioId { get; set; }
public string Culture { get; set; }
public List<ValueModel> Values { get; set; }
public string UserToken { get; set; }
public string ExtResponse { get; set; }
public string PartyName { get; set; }
public string Location { get; set; }
public Guid WebSiteRoutingId { get; set; }
}
ValueModel¶
public class ValueModel
{
public Guid ControlId { get; set; }
public string ResultText { get; set; }
public int? ResultNumber { get; set; }
public DateTime? ResultTime { get; set; }
}
ChatModel¶
public class ChatModel
{
public Guid ChatId { get; set; }
public string AgentName { get; set; }
public Guid? AgentId { get; set; }
public int Counter { get; set; } // Number of messages in conversation
// Identical constants as for Atlantis.iCC.Model.Common.ChatStatusInfo.Status.ST_*
public string Status { get; set; } // Pilot, ICR, Waiting, Alerting, Answered, Closed
public bool IsTyping { get; set; } // Indication that partner is just typing
public List<SentenceModel> Sentences { get; set; }
public string Navigation { get; set; }
public string CoBrowsingData { get; set; }
}
SentenceModel¶
public class SentenceModel
{
public int Counter { get; set; } // pořadové číslo zprávy (od 1), smysluplné jen u zpráv od serveru k prohlížeči
public DateTime TimeSent { get; set; } // čas odeslání, smysluplné jen u zpráv od serveru k prohlížeči
public string PartyName { get; set; } // jak je označena strana konverzace
public bool IsOutbound { get; set; } // true = zpráva od agenta ke klientovi, false = zpráva od klienta k agentovi
public string Text { get; set; } // text konverzace XSS/htmlencoded
public string Location { get; set; } // URL odkud je odesílána zpráva
public bool IsConfirmed { get; set; } // true = zpráva potvrzena protistranou
}
Integration using SimProt/TCP¶
This integration assumes a SimProt Messaging API with formatting structures using JSON and runs through ProServer, with the message broker being the ServiceSync service. On the part of the external application, it can be a specific connector or, for example, a WebSite module. On the client application side of the agent, it can be ReactClient, App.WinClient, etc.
Communication between an external application, the server and the agent’s client for Chat¶
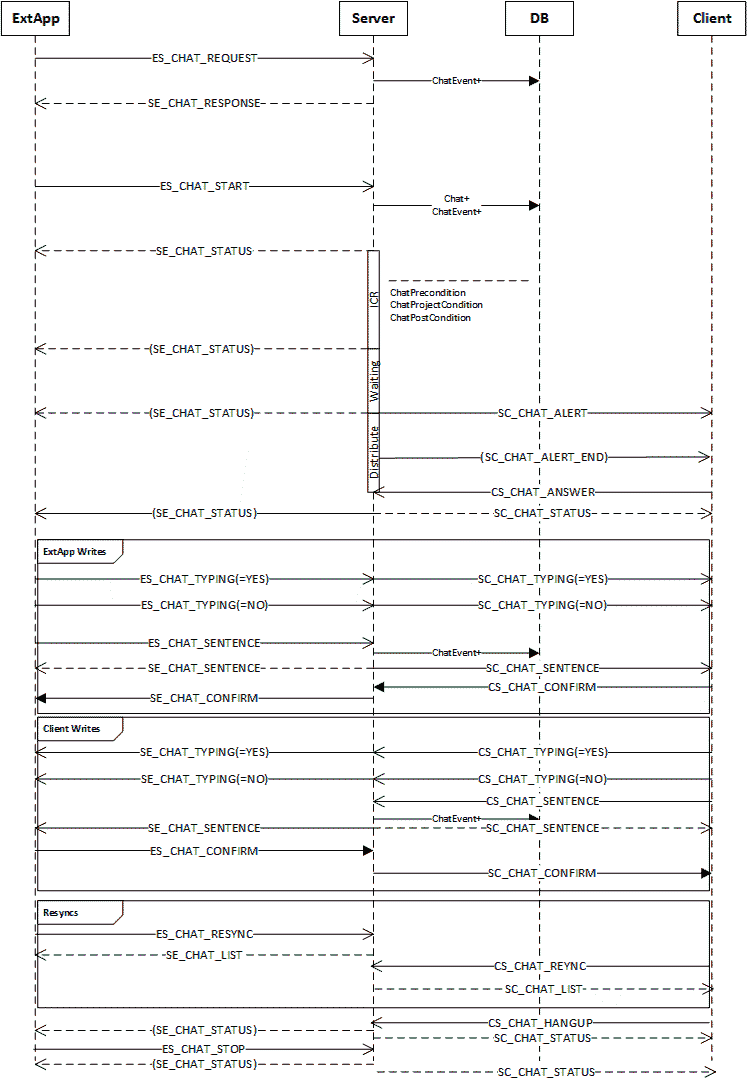
API for external chat applications¶
Important
This interface does not apply to external contact center applications.
You can use the SimProt (TCP and MessagePack) or the .NET Pro.Cti.Proxy.dll and the iCC.Model.Client library, which makes this protocol available as a .NET Standard 2.0 API. The interfaces also serve as integration connectors for instant messaging networks.
The supplied application iCC.Utils.WinChat is used for testing and as a template.
Prologue¶
ES_CHAT_REQUEST [ChatServiceInfo]¶
A request to start a chat. The message transmits the following data:
Peer - The gateway address (corresponds to the ChatGateway.PeerAddress field)
Port - The gateway subaddress (corresponds to the ChatGateway.PeerPort field); optional
TrackingId - If the thread can be identified on the source application side, then the existing ID; otherwise the newly generated ID
PreferredCulture - An ISO2 string
UserToken - A string identifying the user in an external system
ExtResponse - The result of processing by an external logic - a string for CC status calculation
The message is evaluated using ChatGateCondition and by means of the occupancy calculation according to these conditions. The message does not create an entry in the Chat table.
SE_CHAT_RESPONSE [ChatServiceInfo]¶
Information on whether the chat can be started or not, based on ChatGateCondition.
Peer - The gateway address (corresponds to the ChatGateway PeerAddress field)
Port - The gateway subaddress (corresponds to the ChatGateway.PeerPort field); optional
TrackingId - An external identification of the chat (thread) as passed in ES_CHAT_REQUEST
Signal - Information that it is possible to receive a chat
Free - Yes, the chat should be distributed immediately
Busy - Yes, but they will probably wait for a free agent
Closed - No, we are closed
Hidden - No, we are closed and the chat option should not be advertised
ScenarioModel - The structure of the expected form at the start of the chat
This information for a given combination of parameters from ES_CHAT_REQUEST can be cached for some time on the part of the external application (~max. tens of seconds).
Start, status and end¶
ES_CHAT_START [ChatServiceInfo]¶
Information that the chat should start. The parameters are used to evaluate ChatPreCondition, ChatProjectCondition. The message creates an entry in the Chat table or searches for an existing one by TrackingId. The associated form is stored in Chat.FormId and is used to consolidate the new form in the ICR (if used).
Peer - The gateway address (corresponds to the ChatGateway PeerAddress field)
Port - The gateway subaddress (corresponds to the ChatGateway.PeerPort field); optional
TrackingId - If the thread can be identified on the source application side, otherwise a newly generated ID
PreferredCulture - An ISO2 string
UserToken - A string identifying the user in an external system
ExtResponse - The result of processing by an external logic - a string for CC status calculation
ResultModel – A structure with associated form data; values in the form can be evaluated using TextMatch
PartyName - The name of the other party as it should be represented in the conversation (given by the connector)
RemoteAddress - The counterparty’s address (given by the connector)
ReturnChatId - An identifier of the existing (outbound) chat if TrackingId is not previously known; in this situation, it is a matter of establishing a conversation on an outbound chat already existing in the FS; used in case the outbound chat is started by an external logic, which receives ChatId of the pre-created outbound chat; the connector must supply this ID in this field in order to identify the inbound chat and the already created outbound chat in FS.
SE_CHAT_STATUS [ChatStatusInfo]¶
An ongoing chat status with ongoing information.
Peer - The gateway address (corresponds to the ChatGateway PeerAddress field)
Port - The gateway subaddress (corresponds to the ChatGateway.PeerPort field); optional
AgentId - A GUID of the agent or machine that is connected
AgentName - A text representation of the conversing entity (agent or machine)
Status - Indicates in which phase the chat currently is.
Pilot - The chat was registered in the DB and is waiting for the conditions to be evaluated.
ICR - An automatic conversation takes place according to the conditions.
Waiting - No free agent available; the system does not converse.
Alerting - The chat is indicated to the agent, the system is waiting for the agent to accept it.
Answered - A conversation with the agent is in progress.
Closed - The chat was terminated either by the agent or by an external party or due to inactivity.
ChatId - An ID of the ongoing chat in the server database
TrackingId - An external identification of the chat (thread) as passed in ES_CHAT_START
Sentences [] - An array of records of the current conversation; this array is present only in response to SE_CHAT_START and SE_CHAT_RESYNC messages.
ES_CHAT_STOP [ChatCommandInfo]¶
Explicit termination request
Peer - The gateway address (corresponds to the ChatGateway PeerAddress field)
Port - The gateway subaddress (corresponds to the ChatGateway.PeerPort field); optional
TrackingId - An external identification of the chat (thread) as passed in ES_CHAT_START
Inbound direction¶
ES_CHAT_TYPING [ChatCommandInfo]¶
Information that the external party is “writing” or “not writing”.
Peer - The gateway address (corresponds to the ChatGateway PeerAddress field)
Port - The gateway subaddress (corresponds to the ChatGateway.PeerPort field); optional
TrackingId - An external identification of the chat (thread) as passed in ES_CHAT_START
IsTyping - true = the external party is writing, false = the external party is not writing
ES_CHAT_SENTENCE [ChatSentenceInfo]¶
A text message sent from an external application to the server. After sending, the application should wait up to 20 seconds and include the message in the conversation report as sent only once SE_CHAT_SENTENCE is returned. Otherwise (when the message sent to it does not return within the time limit using SE_CHAT_SENTENCE), it should consider the sending as unsuccessful and either automatically retry or leave the retry up to the user.
Peer - The gateway address (corresponds to the ChatGateway PeerAddress field)
Port - The gateway subaddress (corresponds to the ChatGateway.PeerPort field); optional
TrackingId - An external identification of the chat (thread) as passed in ES_CHAT_START
ID - A unique message ID; under this ID it will be listed in the DB (without any uniqueness check, max. 64 characters).
PartyName - The name of the other party as it should be represented in the conversation (given by the connector)
Text - The text of the transmitted message
TimeSent - The time of sending as specified by the other party
IsOutbound - Not used in ES_CHAT_SENTENCE (it is left as “false”); otherwise, it is a direction indication (true = from the client to the extapp, false = from extapp to the client).
Counter - Not used (left as 0); otherwise, it is the sequence number of the message (corresponds to the order in the Sentences field for SE_CHAT_STATUS, numbered from one).
ConfirmedTime - The time of message delivery confirmation to the other party; NULL means not yet confirmed.
Model - The message type (“channel”) - method of interpretation
M_Sentence (0) - A message composed by a person, displayed in a conversation
M_TechNote (2) - An automatically generated message, displayed in a conversation
M_TechPanel (4) - An automatically generated message, displayed statically (last only)
M_Navigation (6) - Information about the counterparty’s navigation (typically a support for WebChat) - URL
M_CoBrowsingData (8) - Data from the counterparty (typically a support for WebChat); the data structure and format depends on the using application.
SE_CHAT_SENTENCE [ChatSentenceInfo]¶
Confirm that the ES_CHAT_SENTENCE message has reached the message broker. It is identical to the sent message but has IsOutbound=false, Counter - according to the sequence number. Based on this message, the application should only display the message in the conversation report (“Observer” behavior pattern).
SE_CHAT_CONFIRM [ChatCommandInfo]¶
A confirmation that the sent message has been delivered to the counterparty.
Peer - The gateway address (corresponds to the ChatGateway PeerAddress field)
Port - The gateway subaddress (corresponds to the ChatGateway.PeerPort field); optional
TrackingId - An external identification of the chat (thread) as passed in ES_CHAT_START
ID - A unique GUID of the message being confirmed
Outbound direction¶
SE_CHAT_TYPING [ChatCommandInfo]¶
Information that the internal party is “writing” or “not writing”.
Peer - The gateway address (corresponds to the ChatGateway PeerAddress field)
Port - The gateway subaddress (corresponds to the ChatGateway.PeerPort field); optional
TrackingId - An external identification of the chat (thread) as passed in ES_CHAT_START
IsTyping - true = The internal party is writing, false = the external party is not writing
SE_CHAT_SENTENCE [ChatSentenceInfo]¶
The text message sent from the internal client from the server.
Peer - The gateway address (corresponds to the ChatGateway PeerAddress field)
Port - The gateway subaddress (corresponds to the ChatGateway.PeerPort field); optional
TrackingId - An external identification of the chat (thread) as passed in ES_CHAT_START
ID - A unique GUID of the message in a text form; under this ID it will be listed in the DB (without a uniqueness check).
PartyName - The name of the other party as it should be represented in the conversation (given by the connector)
Text - The text of the transmitted message
TimeSent - The time of sending as specified by the other party
IsOutbound - Direction indication (true = from the client to the extapp)
Counter - The message sequence number (corresponds to the order in the Sentences field for SE_CHAT_STATUS)
ConfirmedTime - The time of message delivery confirmation to the other party; NULL means not yet confirmed.
Model - The message type (“channel”) - method of interpretation
M_Sentence (0) - A message composed by a person, displayed in a conversation
M_TechNote (2) - An automatically generated message, displayed in a conversation
M_TechPanel (4) - An automatically generated message, displayed statically (last only)
M_Navigation (6) - Information about the counterparty’s navigation (typically a support for WebChat) - URL
M_CoBrowsingData (8) - Data from the counterparty (typically a support for WebChat); the data structure and format depends on the using application.
ES_CHAT_CONFIRM [ChatCommandInfo]¶
A confirmation that the sent message has been delivered.
Peer - The gateway address (corresponds to the ChatGateway PeerAddress field)
Port - The gateway subaddress (corresponds to the ChatGateway.PeerPort field); optional
TrackingId - An external identification of the chat (thread) as passed in ES_CHAT_START
ID - A unique GUID of the message being confirmed
Recovery from interruption¶
ES_CHAT_RESYNC [ChatCommandInfo]¶
A request to send complete information about the status of all chats for a given gateway (connector). The answer is the SE_CHAT_LIST message.
Peer - The gateway address (corresponds to the ChatGateway PeerAddress field)
Port - The gateway subaddress (corresponds to the ChatGateway.PeerPort field); optional
SE_CHAT_LIST [ChatStatusInfo[]]¶
A list of communications that are active for the given connector. The Sentences field is left blank.
Peer - The gateway address (corresponds to the ChatGateway PeerAddress field)
Port - The gateway subaddress (corresponds to the ChatGateway.PeerPort field); optional
AgentId - A GUID of the agent or machine that is connected
AgentName - A text representation of the conversing entity (agent or machine)
Status - An indication of what phase the chat is in - Pilot, ICR, Queue, Alerting, Answered, ClosedByAgent, ClosedByExtApp
ChatId - An ID of the ongoing chat in the server database
TrackingId - An external identification of the chat (thread) as passed in ES_CHAT_START
ES_CHAT_STATUS [ChatStatusInfo]¶
A request to send a complete chat status information. The response is SE_CHAT_STATUS.
Peer - The gateway address (corresponds to the ChatGateway PeerAddress field)
Port - The gateway subaddress (corresponds to the ChatGateway.PeerPort field); optional
TrackingId - An external identification of the chat (thread) as passed in ES_CHAT_START
Handling forms¶
ES_SCENARIO_LOAD [CommandInfo]¶
A request for a form scenario to be sent. In the Id parameter is the ID of the required scenario, in the Reference parameter is the ISO name of the localization language.
SE_SCENARIO_MODEL [ScenarioInfo]¶
The returned scenario structure.
ES_SCENARIO_SAVE [ScenarioResultInfo]¶
A request and form data to be saved.
Registers¶
The registers are transmitted in the beginning and then periodically according to the web.config parameter of DatabaseCaching. These messages are used for transmission.
ES_WS_ROUTING [string]¶
A request to send a table of routing rules. The parameter is an address of the gateway to which the data is to be sent.
SE_WS_ROUTING [WebSiteRoutingInfo[]]¶
A list of routing rules.
ES_WS_LITERALS_GET [LiteralLookupInfo]¶
A request to send a group from the localization table; only the following parameters are filled in:
LiteralGroup - Group number (for WebSite it is 102)
Culture - An ISO name of the localization language (e.g. “en-US”); if it is NULL, it returns all available languages.
Peer - An address of the gateway to which the data is to be sent.
SE_WS_LITERALS_INFO [LiteralLookupInfo[]]¶
The localization table.
An API for internal chat applications¶
This API page relates to a person inside the contact center (agent). Integration on this interface is possible although indirect integration can be assumed by extending the iCC.App.WinClient application with custom functions.
Start, status and end¶
SC_CHAT_ALERT [ChatStatusInfo]¶
A request for the agent to take over a chat.
Peer - A chat workplace number (corresponds to the Workplace Number field)
ChatId - An ID of the ongoing chat in the server database
AgentId - A GUID of the agent or machine that is connected
AgentName - A text representation of the conversing entity (agent or machine)
Status - Indicates in which phase the chat currently is.
Pilot - The chat was registered in the DB and is waiting for the conditions to be evaluated.
ICR - An automatic conversation takes place according to the conditions.
Waiting - No free agent available; the system does not converse.
Alerting - The chat is indicated to the agent, the system is waiting for the agent to accept it.
Answered - A conversation with the agent is in progress.
Closed - The chat was terminated either by the agent or by an external party or due to inactivity.
Sentences [] - An array of records of the current conversation; this field is only present as a response to the CS_CHAT_ANSWER, CS_CHAT_HANGUP and CS_CHAT_RESYNC messages.
Info - See below
The message transmits additional data in the Info field:
Peer - A chat workplace number (corresponds to the Workplace Number field)
TimeStamp - The UTC time when the information was sent.
EnqueueingTimeUtc - The UTC time when the distribution started.
ChatId - The chat GUID
ChatType - The chat channel in FS (BusinessIM, SocialIM, WebIM)
RemotePartyName - The counterparty’s name (according to the connector)
RemoteAddress - The counterparty’s address (if given by the connector)
WorkplaceNumber - The workplace number
WorkplaceId - The workplace ID
AgentId - The agent ID
ProjectId - The project ID
LanguageId - The language ID
ProjectName - The project name
LanguageName - The language name
WaitingTime - The duration of waiting in the queue and before distribution (in seconds)
MaxSignalDuration - The maximum time of chat signaling with the agent
ChatGatewayName - A name of the inbound gateway
ContactId - The contact ID
ContactName - The contact name
Scenarios - A list of all associated form data, including the default form
CS_CHAT_ANSWER [ChaCommandInfo]¶
Information that the agent has taken over the chat.
Peer - A chat workplace number (corresponds to the Workplace Number field)
ChatId - The chat GUID
AgentId - The agent ID
SC_CHAT_ALERT_END [ChaCommandInfo]¶
Information that the chat has been transferred elsewhere (termination only for the agent) after exceeding the maximum signaling time.
Peer - A chat workplace number (corresponds to the Workplace Number field)
ChatId - The chat GUID
AgentId - The agent ID
CS_CHAT_HANGUP [ChaCommandInfo]¶
Information that the agent has rejected or terminated the chat.
Peer - A chat workplace number (corresponds to the Workplace Number field)
ChatId - The chat GUID
AgentId - The agent ID
SC_CHAT_STATUS [ChatStatusInfo]¶
An ongoing chat status with ongoing information.
Peer - A chat workplace number (corresponds to the Workplace Number field)
ChatId - An ID of the ongoing chat in the server database
AgentId - A GUID of the agent or machine that is connected
AgentName - A text representation of the conversing entity (agent or machine)
Status - An indication of what phase the chat is in - Pilot, ICR, Queue, Alerting, Answered, ClosedByAgent, ClosedByExtApp
Sentences [] - An array of records of the current conversation; this field is only present as a response to the CS_CHAT_ANSWER, CS_CHAT_HANGUP and CS_CHAT_RESYNC messages.
Info - The same information as for SC_CHAT_ALERT
Inbound direction¶
SC_CHAT_TYPING [ChaCommandInfo]¶
Information that the external party is “writing” or “not writing”.
Peer - A chat workplace number (corresponds to the Workplace Number field)
ChatId - The chat GUID
AgentId - The agent ID
IsTyping - true = the external party is writing, false = the external party is not writing
SC_CHAT_SENTENCE [ChatSentenceInfo]¶
A text message sent from the server to the internal client
Peer - A chat workplace number (corresponds to the Workplace Number field)
ChatId - The chat GUID
ID - A unique GUID of the message; under this ID it will be listed in the DB (without a uniqueness check).
PartyName - The name of the other party as it should be represented in the conversation (given by the connector)
Text - The text of the transmitted message
TimeSent - The time of sending as specified by the other party
IsOutbound - A direction indication (false = from extapp to the client)
Counter - The message sequence number (corresponds to the order in the Sentences field for SC_CHAT_STATUS)
CS_CHAT_CONFIRM [ChaCommandInfo]¶
A confirmation that the sent message has been delivered.
Peer - A chat workplace number (corresponds to the Workplace Number field)
ChatId - The chat GUID
ID - A unique GUID of the message being confirmed
Outbound direction¶
CS_CHAT_TYPING [ChaCommandInfo]¶
Information that the internal party is “writing” or “not writing”.
Peer - A chat workplace number (corresponds to the Workplace Number field)
ChatId - The chat GUID
IsTyping - true = The internal party is writing, false = the external party is not writing
CS_CHAT_SENTENCE [ChatSentenceInfo]¶
A text messagesent from the internal client to the server. After sending, the application should wait up to 20 sec and include the message in the conversation report as sent only on the basis of returning SC_CHAT_SENTENCE.
Peer - A chat workplace number (corresponds to the Workplace Number field)
ChatId - The chat GUID
ID - A unique GUID of the message; under this ID it will be listed in the DB (without a uniqueness check).
PartyName - The name of the counterparty as it should be represented in the conversation (the agent’s NickName).
Text - The text of the transmitted message
TimeSent - The time of sending as specified by the other party
IsOutbound - Not used (leave true = from the client to extapp); otherwise, it is a direction indication.
Counter - Not used; otherwise, the message sequence number (corresponds to the order in the Sentences field for SC_CHAT_STATUS)
SC_CHAT_SENTENCE [ChatSentenceInfo]¶
Confirm that the CS_CHAT_SENTENCE message has reached the message broker. It is identical to the sent message but has IsOutbound=true, Counter - according to the sequence number. Based on this message, the application should only display the message in the conversation report (“Observer” behavior pattern).
CS_CHAT_CONFIRM [ChatCommandInfo]¶
A confirmation that the sent message has been delivered.
Peer - A chat workplace number (corresponds to the Workplace Number field)
ChatId - The chat GUID
ID - A unique GUID of the message being confirmed
Recovery from interruption¶
CS_CHAT_RESNYC [ChatCommandInfo]¶
A request to send complete information about the status of all chats for a given internal extension (workplace). The answer is the SC_CHAT_LIST list.
Peer - A chat workplace number (corresponds to the Workplace Number field)
AgentId - The agent ID
SC_CHAT_LIST [ChatStatusInfo[] ]¶
A list of communications that are active for the given connector. The Sentences and the Info fields are left blank.
Peer - A chat workplace number (corresponds to the Workplace Number field)
AgentId - The agent ID
ChatId - The chat GUID
AgentName - A text representation of the conversing entity (agent or machine)
Status - An indication of what phase the chat is in - Pilot, ICR, Queue, Alerting, Answered, ClosedByAgent, ClosedByExtApp
CS_CHAT_STATUS [ChatCommandInfo]¶
A request to send complete chat status information. The response is SC_CHAT_STATUS.
A request to send complete chat status information.
Peer - A chat workplace number (corresponds to the Workplace Number field)
AgentId - The agent ID
ChatId - The chat GUID
Data structures for chat¶
The description of data structures is performed below as a C# class. Serialization is performed to JSON, and PascalCasing members are preserved. Only Properties are transmitted.
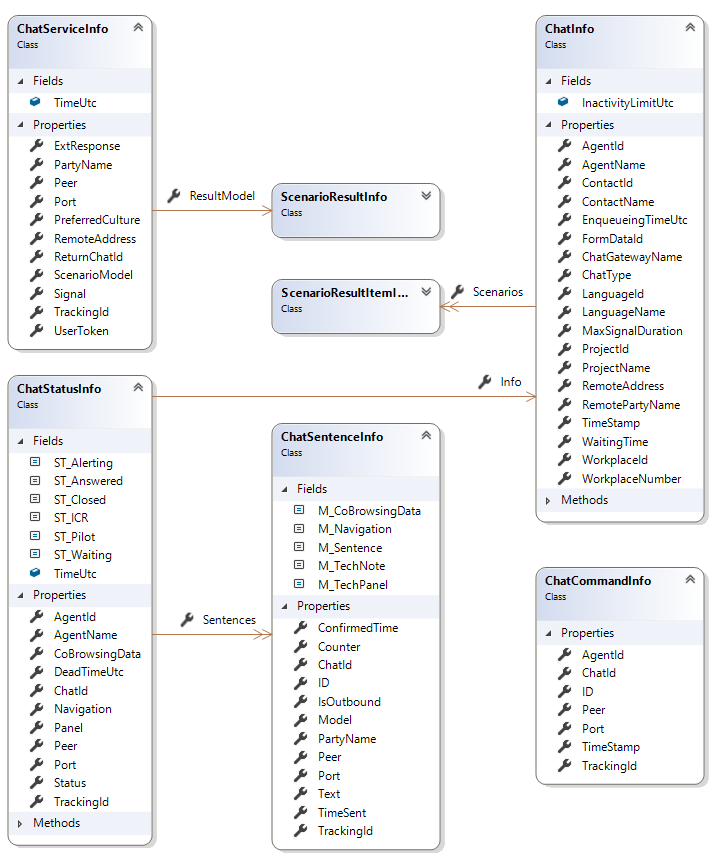
ChatServiceInfo¶
public class ChatServiceInfo
{
public string Peer { get; set; } // ES+SE
public string Port { get; set; } // ES+SE
public string TrackingId { get; set; } // ES+SE
public string PreferredCulture { get; set; } // ES
public string UserToken { get; set; } // ES
public string ExtResponse { get; set; } // ES
public ScenarioResultInfo ResultModel { get; set; } // ES
public string PartyName { get; set; } // ES
public string RemoteAddress { get; set; } // ES
public Guid? ReturnChatId { get; set; } // ES
public string Signal { get; set; } // SE
public ScenarioInfo ScenarioModel { get; set; } // SE
}
ChatStatusInfo¶
public class ChatStatusInfo
{
public string Peer { get; set; } // SE+SC
public string Port { get; set; } // SE
public string TrackingId { get; set; } // SE
public Guid ChatId { get; set; } // SC
public string Status { get; set; }
// SE+SC: Pilot, ICR, Waiting, Alerting, Answered, Closed
public string AgentName { get; set; } // SE
public Guid? AgentId { get; set; } // SE
public List<ChatSentenceInfo> Sentences { get; set; } // SE+SC
public ChatInfo Info { get; set; } // SC
public ChatSentenceInfo Panel { get; set; } // SE+SC
public ChatSentenceInfo Navigation { get; set; } // SE+SC
public ChatSentenceInfo CoBrowsingData { get; set; } // SE+SC
public DateTime? DeadTimeUtc { get; set; } // SE
}
ChatInfo¶
public class ChatInfo
{
public DateTime TimeStamp { get; set; }
public DateTime EnqueueingTimeUtc { get; set; }
public DateTime? PilotDialTime { get; set; }
public string ChatType { get; set; }
// BusinessIM, SocialIM, WebIM
public string RemotePartyName { get; set; }
public string RemoteAddress { get; set; }
public string WorkplaceNumber { get; set; }
public Guid? WorkplaceId { get; set; }
public Guid? AgentId { get; set; }
public Guid? ProjectId { get; set; }
public Guid? LanguageId { get; set; }
public string ProjectName { get; set; }
public string LanguageName { get; set; }
public string AgentName { get; set; }
public int WaitingTime { get; set; }
public int MaxSignalDuration { get; set; }
public string ChatGatewayName{ get; set; }
public Guid? ContactId { get; set; }
public string ContactName { get; set; }
public List<ScenarioResultItemInfo> Scenarios { get; set; }
public Guid? FormDataId{ get; set; }
}
ScenarioResultItemInfo¶
public class ScenarioResultItemInfo
{
public Guid ScenarioId { get; set; }
public string ScenarioName { get; set; }
public string Activity { get; set; }
public Guid? ResultId { get; set; }
public string RemoteName { get; set; }
public string DisplayName { get; set; }
public DateTime? ResultCreated { get; set; }
public string ShowInList { get; set; }
}
ChatSentenceInfo¶
public class ChatSentenceInfo
{
public string Peer { get; set; } // ES+SE+CS+SC
public string Port { get; set; } // ES+SE
public string TrackingId { get; set; } // ES+SE
public Guid ChatId { get; set; } // CS+SC
public string ID { get; set; } // ES+SE+CS+SC, max. 64 znaků
public string PartyName { get; set; } // ES+SE+CS+SC
public string Text { get; set; } // ES+SE+CS+SC
public DateTime TimeSent { get; set; } // SE+SC
public bool IsOutbound { get; set; } // SE+SC
public int Counter { get; set; } // SE+SC
public DateTime? ConfirmedTime { get; set; } // SE+SC
public int Model { get; set; }
// ES+SC 0=Sentence, 2=TechNote, 4=TechPanel, 6=Navigation, 8=CoBrowsingData
}
ChatCommandInfo¶
public class ChatCommandInfo
{
public string Peer { get; set; }
public string Port { get; set; } // ES+SE
public string TrackingId { get; set; }
public Guid? ChatId { get; set; }
public string ID { get; set; }
public DateTime? TimeStamp { get; set; }
public Guid? AgentId { get; set; }
}
ScenarioResultInfo¶
public class ScenarioResultInfo
{
public Guid ScenarioId { get; set; }
public Guid? ScenarioResultId { get; set; }
public DateTime TimeUtc { get; set; }
public List<ScenarioResultValueInfo> Values { get; set; }
public Guid? AgentId { get; set; }
public Guid? InboundCallId { get; set; }
public Guid? OutboundCallId { get; set; }
public Guid? MessageId { get; set; }
public Guid? IssueId { get; set; }
public Guid? ContactId { get; set; }
public Guid? ChatId { get; set; }
public string Activity { get; set; }
public Guid? WorkflowId { get; set; }
}
ScenarioResultValueInfo¶
public class ScenarioResultValueInfo
{
public Guid ScreenControlId { get; set; }
public int? ResultNumber { get; set; }
public string ResultText { get; set; }
public decimal? ResultNumeric { get; set; }
public DateTime? ResultTime { get; set; }
public string TargetColumn { get; set; }
}
ScenarioInfo¶
public class ScenarioInfo
{
public Guid ScenarioId { get; set; }
public string DisplayName { get; set; }
public List<ScreenInfo> Screens { get; set; }
public Guid? AgentId { get; set; }
public string Culture { get; set; }
}
ScreenInfo¶
public class ScreenInfo
{
public Guid ScreenId { get; set; }
public string DisplayName { get; set; }
public int Rank { get; set; }
public bool FinishButton { get; set; }
public Guid? ConditionScreenControlId { get; set; }
public string ConditionText { get; set; }
public List<ScreenControlInfo> Controls { get; set; }
}
ScreenControlInfo¶
public class ScreenControlInfo
{
public Guid ScreenControlId { get; set; }
public string DisplayName { get; set; }
public string Description { get; set; }
public string TargetColumn { get; set; }
public int Rank { get; set; }
public string ControlType { get; set; }
public int? ControlNumber { get; set; }
public string ControlText { get; set; }
public bool MandatoryField { get; set; }
public List<ScreenControlParameterInfo> Parameters { get; set; }
}
ScreenControlParameterInfo¶
public class ScreenControlParameterInfo
{
public Guid ScreenControlParameterId { get; set; }
public string DisplayName { get; set; }
public int Rank { get; set; }
public int? ResultNumber { get; set; }
public string ResultText { get; set; }
public bool DefaultItem { get; set; }
}
LiteralLookupInfo¶
public class LiteralLookupInfo
{
public short LiteralGroup { get; set; }
public short LiteralValue { get; set; }
public string Culture { get; set; }
public string LiteralText { get; set; }
public string DisplayName { get; set; }
}
WebSiteRoutingInfo¶
public class WebSiteRoutingInfo
{
public Guid WebSiteRoutingId { get; set; }
public string WebApp { get; set; }
public int Rank { get; set; }
public string PageUrlRegex { get; set; }
public string PreferredCulture { get; set; }
public string BrowserRegex { get; set; }
public string UserTokenRegex { get; set; }
public string ClientIpMask { get; set; }
public string TimeMode { get; set; }
public DateTime? TimeFrom { get; set; }
public DateTime? TimeTo { get; set; }
public string TargetElementID { get; set; }
public string WebResponse { get; set; }
public Guid? ScenarioId { get; set; }
public Guid? OutboundListId { get; set; }
public Guid? WorkflowId { get; set; }
public Guid? MessageId { get; set; }
public string LiteralCulture { get; set; }
public int ChatTrackUrl { get; set; }
public bool LogUrl { get; set; }
public string Template { get; set; }
public bool Block { get; set; }
public int? SolicitationTimeOut { get; set; }
public int? SolicitationDuration { get; set; }
public int? HonorRejectPeriod { get; set; }
}
Communication between an external application, the server and the agent’s client for Message¶
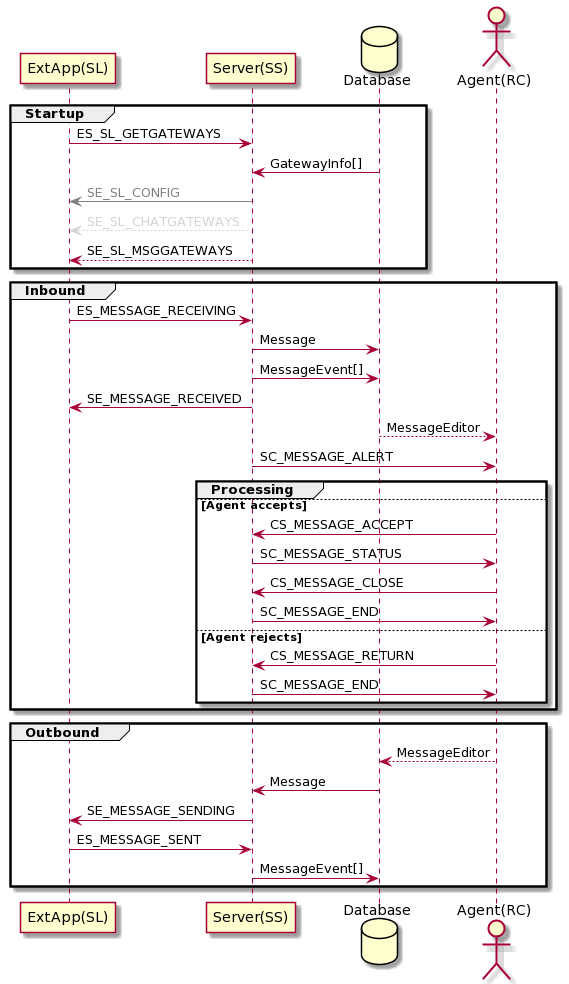
Note
In this section the word “message” is used for any e-mail, SMS or fax message, i.e. the Message
table entity in the database. The abbreviation “MSG” is used for a SimProt protocol unit with a defined structure sent between modules and applications.
API for external messaging applications¶
Note
This interface does not apply to external contact center applications.
You can use the SimProt (TCP and MessagePack) or the .NET Pro.Cti.Proxy.dll and iCC.Model.Client libraries that make this protocol available as a .NET Standard 2.0 API. The interfaces also serve as integration connectors for messaging (Email, SMS, Fax, SocWall).
The supplied application iCC.Utils.WinChat is used for testing and as a template.
Startup¶
The initial dialog that the application uses to find out what gateways are defined and what their configuration is. According to its own settings and according to the connector type specified in Device
, the external application performs its settings and executes the action. Without this information, it cannot send or receive messages.
ES_SL_GETGATEWAYS [string]¶
Request for information about the configuration of message gateways. The MSG transmits a single piece of information: the gateway address (corresponding to the GatewayInfo.PeerAddress
field in Gateway InDevice/OutDevice)
SE_SL_MSGGATEWAYS [ChatGatewayInfo[]]¶
The information about the configuration of message gateways is an array of ChatGatewayInfo structures. The gateways for the inbound direction and the outbound direction are maintained separately.
Id - GUID of the gateway (main data for processing by the external application and the server)
DisplayName - gateway name
PeerAddress - gateway address in MSG routing (corresponds to the ChatGateway PeerAddress field)
PeerPort - not used for message transmission
Channel - indicates the channel from which the gateway processes messages (ChannelIndex)
Direction - gateway direction (‘I’ - inbound, ‘O’ - outbound)
Device - technical configuration of the gateway for the given direction containing the connector type and the subsequent JSON configuration
LanguageId - GUID of the default gateway language
Inbound¶
Inbound message processing by the server using MSG.
ES_MESSAGE_RECEIVING [MessageContentInfo]¶
Inbound message; information from an external application to the server. The following fields are passed in the MSG:
DeliverySystemId - an external message identifier as understood by the external application; it will be stored in the database in this form(max. 80 characters), mandatory item
InReplyToId - if the received message is a reply, then the external identifier of the message to which it replies is given here (used to find the related conversation)
FromField - text information about the sender (formatting should correspond to the Email, SMS, Fax channel); used for display (max. 160 characters)
RemoteAddress - the sender’s address (its format should correspond to the Email, SMS, Fax channel); used for creating a reply; a mandatory item (max. 250 characters)
ToField - text information about the direct recipient (its format should correspond to the Email, SMS, Fax channel); used for display (max. 320 characters)
ToCcField - text information about the indirect recipient (its format should correspond to the Email channel; it is usually not used for SMS, Fax); used for display (max. 320 characters)
ToBccField - text information about the hidden recipient; usually not used for inbound messages (max. 320 characters)
Direction - direction indication; if specified; it should be ‘I’
ReceivedSentTime - indication of the time when the message was received by the interface connector in the UTC time; a mandatory item
DraftTime - indication of the time when the message was by the counterparty in the UTC
SubjectField - message title/name (max. 320 characters)
BodyHtml - message content in HTML formatting; if not supplied, it will be converted from the BodyText version
BodyText - message content as plain text; if not supplied, it will be converted from the BodyHtml version
Culture - message language (if known) in the ISO format (e.g. en-US)
Mark - processing field (an integer); it will be stored in the corresponding database field if specified
SpamLevel - indication of spam detection; n/a - no detection, 0 - no spam, 1 - spam assigned manually, 2 - spam assigned to a contact, 3 - spam detected by external application (screening)
SignatureValidity - indication of digital integrity check; not specified - no check was performed, -1 - the message has no digital signature, 0 - the message has a valid digital signature, positive number - the digital signature of the message contains an error with this number (specific for the connector)
Hierarchy - indication of the message position in the hierarchy - it can be processed by the hierarchy editor according to the channel type
Rating - indication of satisfaction with the message; it will be stored in the corresponding field in the database if specified
GatewayId - the GUID of the gateway through which the message was received must match the set of gateways obtained by SE_SL_MSGGATEWAYS
RequestId - local correlation GUID for the possibility of pairing the MSG of the inbound message with its asynchronous acknowledgement
SE_MESSAGE_RECEIVED [CommandInfo]¶
An inbound message; information from the server to the external application (connector) that the inbound message has been processed. The following fields are passed in the MSG:
MessageId (Id) - GUID of the message as it was entered in the database in the Message table
GatewayId (RefId) - the GUID of the gateway that received the message must match the set of gateways obtained by SE_SL_MSGGATEWAYS (mirrored from ES_MESSAGE_RECEIVING)
RequestId (Reference) - local correlation (id) for the possibility of pairing the MSG acknowledgement with previously sent message content (mirrored from ES_MESSAGE_RECEIVING)
Success (Data) - processing result (true - successfully processed, can be confirmed to the counterparty; false - processing failed, the connector can either try to send the message to the server again after a delay, or can indicate failure to the counterparty)
TimeStamp - time of message acknowledgment on the server (UTC)
Note
The server sends just one response SE_MESSAGE_RECEIVED to ES_MESSAGE_RECEIVING. The connector should wait at least 60 seconds for a response; the server response rate depends on the load.
Outbound¶
Outbound message processing by the connector using MSG.
SE_MESSAGE_SENDING [MessageContentInfo]¶
An outbound message; information from the server to the external application (connector). The following fields are passed in the MSG:
MessageId (Id) - GUID of the message as entered in the database in the Message table; a mandatory item
DeliverySystemId - proposal for the external message identifier under which it is to be registered by the external application; this is how it is stored in the database (max. 80 characters); a mandatory item
FromField - text information about the sender (its format should correspond to the Email, SMS, Fax channel); used for displaying
RemoteAddress - address of the recipient (its format should correspond to the Email, SMS, Fax channel); a mandatory item
ToField - text information about the direct recipient (its format should correspond to the Email, SMS, Fax channel); used for displaying
ToCcField - text information about the indirect recipient (its format should correspond to the Email channel; it is usually not used for SMS, Fax); used for displaying
ToBccField - text information about the hidden recipient (its format should correspond to the Email channel; it is usually not used for SMS, Fax); used for displaying
ExtendedFields - structured information about recipients (its format corresponds to the channel); it can be used for addressing instead of RemoteAddress
Direction - direction indication; if specified; it should be ‘O’
ReceivedSentTime - indication of the time when the message was sent by the server to the interface in the UTC time; a mandatory item
DraftTime - indication of the start time of message writing in the UTC
SubjectField - message title/name (max. 320 characters)
BodyHtml - message content in the HTML format
BodyText - message content as a plain text
Mark - processing field (integer); drawn from the corresponding field in the database
Hierarchy - indication of the message position in the hierarchy - it can be processed by the hierarchy connector according to the channel type
GatewayId - GUID of the gateway through which the message is to leave; corresponds to the set of gateways obtained by SE_SL_MSGGATEWAYS
ES_MESSAGE_SENT [CommandInfo]¶
An outbound message; information from the external application to the server that a message has been sent. The following fields are passed in the MSG:
MessageId (Id) - GUID of the message as it is stored in the database in the Message table (mirrored from SE_MESSAGE_SENDING)
GatewayId (RefId) - GUID of the gateway through which the message was sent; it must match the set of gateways obtained by SE_SL_MSGGATEWAYS (mirrored from SE_MESSAGE_SENDING)
DeliverySystemId (Reference) - the actual external identifier of the message as registered by the external application; this is how it will be stored in the database upon acknowledgment (max. 80 characters); if it is null, the draft from SE_MESSAGE_SENDING is left in the database
Error (Data) - result of processing (null - successfully processed, otherwise - sending failed; error text as it will be stored in the database in the MessageEvent table as the reason of failure)
TimeStamp - indicates the time when the acknowledgement message acknowledgment was sent at the connector (UTC)
Note
The connector has to send just one ES_MESSAGE_SENT response to SE_MESSAGE_SENDING within 90s. If the time limit is not met, the delivery is considered failed and will be retried.
Data structures for messages¶
The description of data structures is performed below as a C# class. Serialization is performed to JSON, and PascalCasing members are preserved. Only Properties are transmitted.
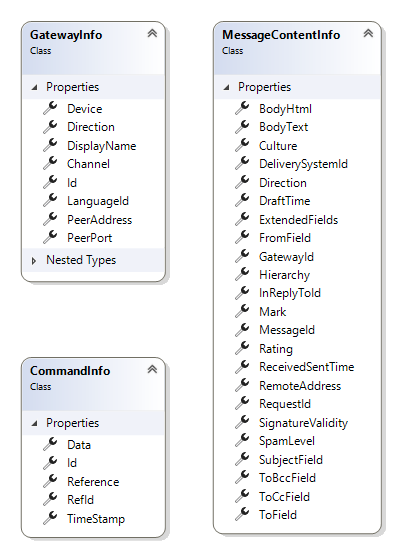
GatewayInfo¶
public class GatewayInfo
{
public string DisplayName { get; set; }
public string PeerAddress { get; set; }
public string PeerPort { get; set; }
public string Device { get; set; }
public ChannelIndex Channel { get; set; }
public string Direction { get; set; }
public Guid? LanguageId { get; set; }
public Guid Id { get; set; }
}
MessageContentInfo¶
public class MessageContentInfo
{
// Message identification
public string DeliverySystemId { get; set; } // SE+ES
public string InReplyToId { get; set; } // ES
public Guid? MessageId { get; set; } // SE+ES - correlation outbound
public string RequestId { get; set; } // SE+ES - correlation inbound
public Guid GatewayId { get; set; } // SE+ES - which GW message belongs to
// Addressing
public string FromField { get; set; } // SE+ES
public string RemoteAddress { get; set; } // SE+ES
public string ToField { get; set; } // SE+ES
public string ToCcField { get; set; } // SE+ES
public string ToBccField { get; set; } // SE+ES
public string Direction { get; set; } // SE+ES
public string ExtendedFields { get; set; } // SE - optionaly for Send and get only
// Timimg
public DateTime ReceivedSentTime { get; set; } // SE+ES UTC
public DateTime? DraftTime { get; set; } // SE+ES UTC
// Content
public string SubjectField { get; set; } // SE+ES - will be XSSed
public string BodyHtml { get; set; } // SE+ES - will be XSSed, if NULL converted from BodyText
public string BodyText { get; set; } // SE+ES - will be XSSed, if NULL converted from BodyHtml
// Metacontent
public string Culture { get; set; } // SE+ES - hint for Language if available from connector
// Metadata
public int? Mark { get; set; } // SE+ES
public int? SpamLevel { get; set; } // SE+ES
public int? SignatureValidity { get; set; } // SE+ES
public int? Hierarchy { get; set; } // SE+ES
public short? Rating { get; set; } // SE+ES
}
CommandInfo¶
public class CommandInfo
{
public Guid? Id { get; set; }
public Guid? RefId { get; set; }
public DateTime? TimeStamp { get; set; }
public string Reference { get; set; }
public string Data { get; set; }
}